c语言 五子棋代码 用栈实现悔棋功能
时间: 2023-08-12 13:34:02 浏览: 58
好的,下面是使用栈实现悔棋功能的五子棋代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define ROW 15
#define COL 15
#define CHESS_NUM 5
typedef struct Point {
int x;
int y;
} Point;
typedef struct Stack {
Point data;
struct Stack *next;
} Stack;
// 初始化棋盘
void initBoard(char board[][COL]) {
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
board[i][j] = '+';
}
}
}
// 打印棋盘
void printBoard(char board[][COL]) {
printf(" ");
for (int i = 0; i < COL; i++) {
printf("%d ", i);
}
printf("\n");
for (int i = 0; i < ROW; i++) {
printf("%d ", i);
for (int j = 0; j < COL; j++) {
printf("%c ", board[i][j]);
}
printf("\n");
}
printf("\n");
}
// 判断是否胜利
int isWin(char board[][COL], char chess) {
int count = 0;
// 判断行
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
if (board[i][j] == chess) {
count++;
if (count == CHESS_NUM) {
return 1;
}
} else {
count = 0;
}
}
count = 0;
}
// 判断列
for (int i = 0; i < COL; i++) {
for (int j = 0; j < ROW; j++) {
if (board[j][i] == chess) {
count++;
if (count == CHESS_NUM) {
return 1;
}
} else {
count = 0;
}
}
count = 0;
}
// 判断左上到右下的斜线
for (int i = 0; i < ROW - CHESS_NUM + 1; i++) {
for (int j = 0; j < COL - CHESS_NUM + 1; j++) {
for (int k = 0; k < CHESS_NUM; k++) {
if (board[i+k][j+k] == chess) {
count++;
if (count == CHESS_NUM) {
return 1;
}
} else {
count = 0;
}
}
count = 0;
}
}
// 判断左下到右上的斜线
for (int i = CHESS_NUM - 1; i < ROW; i++) {
for (int j = 0; j < COL - CHESS_NUM + 1; j++) {
for (int k = 0; k < CHESS_NUM; k++) {
if (board[i-k][j+k] == chess) {
count++;
if (count == CHESS_NUM) {
return 1;
}
} else {
count = 0;
}
}
count = 0;
}
}
return 0;
}
// 入栈操作
void push(Stack **top, Point data) {
Stack *node = (Stack *)malloc(sizeof(Stack));
node->data = data;
node->next = *top;
*top = node;
}
// 出栈操作
Point pop(Stack **top) {
if (*top == NULL) {
printf("栈为空,不能执行出栈操作!\n");
exit(1);
}
Point data = (*top)->data;
Stack *temp = *top;
*top = (*top)->next;
free(temp);
return data;
}
// 初始化栈
void initStack(Stack **top) {
*top = NULL;
}
int main() {
char board[ROW][COL];
Point lastStep;
Stack *stack;
initBoard(board);
initStack(&stack);
printBoard(board);
while (1) {
int x, y;
printf("请输入坐标(x,y):");
scanf("%d,%d", &x, &y);
if (x < 0 || x >= ROW || y < 0 || y >= COL) {
printf("输入坐标超出范围,请重新输入!\n");
continue;
}
if (board[x][y] != '+') {
printf("此处已有棋子,请重新输入!\n");
continue;
}
board[x][y] = 'O';
lastStep.x = x;
lastStep.y = y;
push(&stack, lastStep);
printBoard(board);
if (isWin(board, 'O')) {
printf("你赢了!\n");
break;
}
printf("电脑下棋...\n");
while (1) {
int row = rand() % ROW;
int col = rand() % COL;
if (board[row][col] == '+') {
board[row][col] = 'X';
lastStep.x = row;
lastStep.y = col;
push(&stack, lastStep);
printBoard(board);
if (isWin(board, 'X')) {
printf("电脑赢了!\n");
break;
}
break;
}
}
if (isWin(board, 'X')) {
printf("电脑赢了!\n");
break;
}
char c;
printf("是否悔棋?(y/n)");
scanf(" %c", &c);
if (c == 'y' || c == 'Y') {
Point p = pop(&stack);
board[p.x][p.y] = '+';
p = pop(&stack);
board[p.x][p.y] = '+';
printBoard(board);
}
}
return 0;
}
```
以上代码实现了五子棋的基本功能,同时使用了栈来实现悔棋功能。在每次下棋时,将该步骤的坐标入栈,当需要悔棋时,出栈两次,将棋盘上对应位置的棋子清空即可。
相关推荐
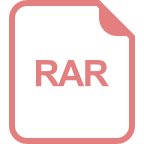
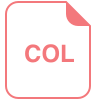
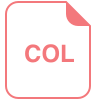












