C语言五子棋用栈实现悔棋
时间: 2023-08-20 08:30:50 浏览: 64
好的,我来为你解答。在C语言中,我们可以用栈来实现悔棋功能。具体实现方法如下:
1. 定义一个结构体来表示棋盘上的一个棋子,包括棋子的位置和颜色等信息。
```
struct Chess {
int x; // 棋子的横坐标
int y; // 棋子的纵坐标
int color; // 棋子的颜色,0表示黑棋,1表示白棋
};
```
2. 定义一个栈来存储棋子的信息。
```
#define MAX_SIZE 100 // 栈的最大容量
struct Stack {
struct Chess data[MAX_SIZE]; // 存储棋子的数组
int top; // 栈顶指针
};
```
3. 实现悔棋功能,即将最后一个下的棋子从棋盘上删除,并将其信息压入栈中。
```
void undo(struct ChessBoard *board, struct Stack *stack) {
if (stack->top == 0) {
printf("已经悔到最后一步了,不能再悔棋了!\n");
return;
}
// 从栈中弹出最后一个棋子的信息
struct Chess chess = stack->data[--stack->top];
// 从棋盘上删除该棋子
board->board[chess.x][chess.y] = -1;
// 打印悔棋信息
printf("悔棋成功!已经将 %s 的 %d 行 %d 列的棋子删除。\n",
chess.color == 0 ? "黑方" : "白方", chess.x, chess.y);
}
```
4. 实现落子功能,即将新下的棋子信息添加到棋盘上,并将其信息压入栈中。
```
void playChess(struct ChessBoard *board, struct Stack *stack, int x, int y, int color) {
// 检查是否越界
if (x < 0 || x >= board->size || y < 0 || y >= board->size) {
printf("位置越界,请重新输入!\n");
return;
}
// 检查该位置是否已经有棋子
if (board->board[x][y] != -1) {
printf("该位置已经有棋子,请重新输入!\n");
return;
}
// 在棋盘上添加新棋子
board->board[x][y] = color;
// 将新棋子的信息压入栈中
struct Chess chess;
chess.x = x;
chess.y = y;
chess.color = color;
stack->data[stack->top++] = chess;
// 打印落子信息
printf("%s 在 %d 行 %d 列落子成功!\n", color == 0 ? "黑方" : "白方", x, y);
}
```
以上就是用栈实现悔棋的具体步骤,希望能对你有所帮助。
相关推荐
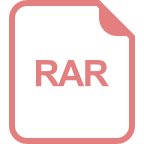














