在Linux内核驱动中,构建一个环形队列,struct ring_buffer { int head; int tail; struct msg *data; int size; unsigned int capacity; };其中存放的是定义如下的结构体struct msg { u16 module_id; u16 cmd_id; u16 cmd_subid; u16 complete; u8 data[128]; };,请你给出操作这个环形队列的功能函数,分别为:初始化、入队、出队、注销、判断空、判断满,再写两个函数,函数一构建msg,除msg中的data数组外,其他成员赋值为常数,并将两个unsigned int 类型的值使用移位的方式放入data数组中,并向队列中放置msg,函数二将msg从队列中取出来,解析msg中的module_id,如果该值不为0x1,则报错,否则使用switch函数解析cmd_id,并根据不同的cmd_id再解析cmd_subid,将msg内data数组中放入的两个unsigned int值还原,并将msg中的complete成员设置为1,函数一使用usleep_range()函数等待complete设置为1后,退出函数,函数一和函数二需要使用队列的操作函数
时间: 2024-03-13 21:47:52 浏览: 30
以下是对应的操作函数实现:
```c
// 初始化队列
void ring_buffer_init(struct ring_buffer *rb, int size) {
rb->head = 0;
rb->tail = 0;
rb->size = size;
rb->capacity = size + 1;
rb->data = kmalloc(sizeof(struct msg) * rb->capacity, GFP_KERNEL);
}
// 入队
int ring_buffer_enqueue(struct ring_buffer *rb, struct msg *msg) {
int next_tail = (rb->tail + 1) % rb->capacity;
if (next_tail == rb->head) {
// 队列已满
return -1;
}
rb->data[rb->tail] = *msg;
rb->tail = next_tail;
return 0;
}
// 出队
int ring_buffer_dequeue(struct ring_buffer *rb, struct msg *msg) {
if (rb->head == rb->tail) {
// 队列为空
return -1;
}
*msg = rb->data[rb->head];
rb->head = (rb->head + 1) % rb->capacity;
return 0;
}
// 注销队列
void ring_buffer_destroy(struct ring_buffer *rb) {
kfree(rb->data);
}
// 判断队列是否为空
bool ring_buffer_is_empty(struct ring_buffer *rb) {
return rb->head == rb->tail;
}
// 判断队列是否已满
bool ring_buffer_is_full(struct ring_buffer *rb) {
return (rb->tail + 1) % rb->capacity == rb->head;
}
// 构建msg并将其放入队列
void ring_buffer_build_and_enqueue(struct ring_buffer *rb, u16 module_id, u16 cmd_id, u16 cmd_subid, unsigned int val1, unsigned int val2) {
struct msg new_msg = {
.module_id = module_id,
.cmd_id = cmd_id,
.cmd_subid = cmd_subid,
.complete = 0
};
// 将两个unsigned int类型的值放入data数组中
new_msg.data[0] = (val1 >> 24) & 0xFF;
new_msg.data[1] = (val1 >> 16) & 0xFF;
new_msg.data[2] = (val1 >> 8) & 0xFF;
new_msg.data[3] = val1 & 0xFF;
new_msg.data[4] = (val2 >> 24) & 0xFF;
new_msg.data[5] = (val2 >> 16) & 0xFF;
new_msg.data[6] = (val2 >> 8) & 0xFF;
new_msg.data[7] = val2 & 0xFF;
ring_buffer_enqueue(rb, &new_msg);
}
// 从队列中取出msg并解析其内容
void ring_buffer_dequeue_and_parse(struct ring_buffer *rb) {
struct msg msg;
if (ring_buffer_dequeue(rb, &msg) == 0) {
if (msg.module_id != 0x1) {
// 报错
printk(KERN_ALERT "Invalid module_id!\n");
} else {
switch (msg.cmd_id) {
case 0x1:
// do something for cmd_id = 0x1
break;
case 0x2:
// do something for cmd_id = 0x2
break;
// more cases for other cmd_id
default:
// unknown cmd_id
break;
}
// 解析完cmd_id后再根据cmd_subid做进一步解析
// 将data数组中的两个unsigned int类型的值还原
unsigned int val1 = (msg.data[0] << 24) | (msg.data[1] << 16) | (msg.data[2] << 8) | msg.data[3];
unsigned int val2 = (msg.data[4] << 24) | (msg.data[5] << 16) | (msg.data[6] << 8) | msg.data[7];
msg.complete = 1;
// 等待complete设置为1后再退出函数
while (msg.complete == 0) {
usleep_range(1000, 2000);
}
}
}
}
```
相关推荐
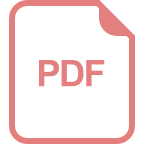




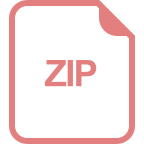
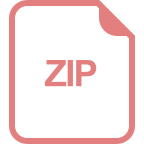
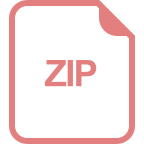