'kernel': ['linear', 'poly', 'rbf', 'sigmoid']}
时间: 2024-04-03 14:29:51 浏览: 168
在机器学习中,'kernel'是一个重要的参数,用于定义支持向量机(SVM)模型中的核函数。核函数是一种将输入数据映射到高维特征空间的函数,它可以帮助我们在非线性问题上进行线性分类或回归。
以下是几种常见的核函数类型:
1. 线性核函数(linear):线性核函数在特征空间中直接进行线性分类或回归,适用于线性可分的问题。
2. 多项式核函数(poly):多项式核函数通过将输入数据映射到高维空间,并使用多项式函数来进行分类或回归。它可以处理一些非线性问题。
3. 径向基函数核(RBF):径向基函数核是一种常用的非线性核函数,它通过将输入数据映射到无穷维的特征空间,并使用高斯分布来进行分类或回归。它适用于各种非线性问题。
4. sigmoid核函数(sigmoid):sigmoid核函数通过将输入数据映射到高维空间,并使用sigmoid函数来进行分类或回归。它适用于二分类问题。
这些不同类型的核函数可以根据数据的特点和问题的需求进行选择。在使用SVM模型时,选择合适的核函数对于模型的性能和准确性非常重要。
相关问题
poly rbf sigmoid 给出这三个代码的示例
以下是三个常见的机器学习算法的示例代码:
1. 多项式回归(Polynomial Regression):
```python
from sklearn.preprocessing import PolynomialFeatures
from sklearn.linear_model import LinearRegression
# 创建多项式特征
poly_features = PolynomialFeatures(degree=2)
X_poly = poly_features.fit_transform(X)
# 创建线性回归模型
model = LinearRegression()
# 拟合多项式回归模型
model.fit(X_poly, y)
# 进行预测
y_pred = model.predict(X_poly)
```
2. 径向基函数(Radial Basis Function, RBF):
```python
from sklearn.svm import SVC
# 创建SVC模型,使用RBF核函数
model = SVC(kernel='rbf')
# 拟合SVC模型
model.fit(X, y)
# 进行预测
y_pred = model.predict(X)
```
3. Sigmoid函数:
```python
import numpy as np
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 示例用法
x = np.array([0, 1, 2, 3, 4])
print(sigmoid(x))
```
以上示例代码中,多项式回归使用了`sklearn`库的`PolynomialFeatures`和`LinearRegression`类;径向基函数使用了`sklearn`库的`SVC`类;sigmoid函数的示例代码与前文提供的代码示例相同。
请注意,这些示例代码仅用于演示目的,实际应用时可能需要根据具体情况进行参数调整和数据预处理。
create a Pipeline that uses SVC (instead of LinearSVC), and applies GridSearchCV to tune the following hyperparameters: C: From 1 to 10 as before kernel: 'linear', 'poly', 'rbf', 'sigmoid' decision_function_shape: 'ovr', 'ovo'
Certainly! Here's an example code snippet that creates a pipeline using SVC and applies GridSearchCV to tune the specified hyperparameters:
```python
from sklearn.pipeline import Pipeline
from sklearn.svm import SVC
from sklearn.datasets import load_iris
from sklearn.model_selection import GridSearchCV
from sklearn.preprocessing import StandardScaler
# Load the Iris dataset
data = load_iris()
X = data.data
y = data.target
# Create a pipeline with StandardScaler and SVC
pipeline = Pipeline([
('scaler', StandardScaler()),
('svc', SVC())
])
# Define the hyperparameters to tune
parameters = {
'svc__C': range(1, 11),
'svc__kernel': ['linear', 'poly', 'rbf', 'sigmoid'],
'svc__decision_function_shape': ['ovr', 'ovo']
}
# Create the GridSearchCV object
grid_search = GridSearchCV(pipeline, parameters)
# Fit the pipeline on the data using GridSearchCV
grid_search.fit(X, y)
# Print the best parameters and best score
print("Best Parameters:", grid_search.best_params_)
print("Best Score:", grid_search.best_score_)
```
Make sure you have the necessary packages installed (e.g., scikit-learn) and adjust the code according to your specific requirements. This code will perform a grid search over the specified hyperparameters and output the best parameters and best score found during the search.
阅读全文
相关推荐
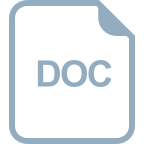
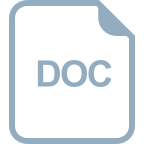
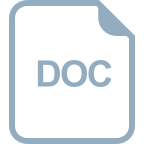













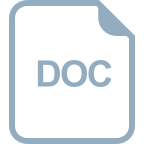