ros noetic moveit 将点云话题的所有点云经过open3d三角化后为一个整体后转换成对应点云位姿的环境scene的python函数
时间: 2024-03-09 15:45:20 浏览: 133
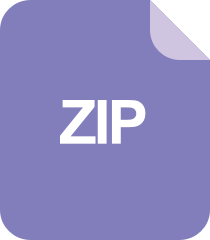
point3d 点云三维重建

您,针对您的问题,我可以提供以下的 python 函数实现:
```python
import open3d as o3d
import rospy
from moveit_msgs.msg import PlanningScene, ObjectColor
from geometry_msgs.msg import PoseStamped
from sensor_msgs.msg import PointCloud2
from tf2_sensor_msgs.tf2_sensor_msgs import do_transform_cloud
from tf2_geometry_msgs import do_transform_pose
def add_pointcloud_to_scene(pointcloud_topic, pointcloud_frame, scene_publisher, color=[0, 0, 0]):
"""
将指定 topic 的点云转换为场景中的物体并发布到 PlanningScene 中
:param pointcloud_topic: 点云的 topic
:param pointcloud_frame: 点云的坐标系
:param scene_publisher: PlanningScene 的发布者
:param color: 物体的颜色,RGB 值的数组,默认为黑色
"""
# 订阅指定 topic 的点云
rospy.loginfo("Waiting for point cloud message on topic {}...".format(pointcloud_topic))
pointcloud_msg = rospy.wait_for_message(pointcloud_topic, PointCloud2)
# 将点云转换成 open3d 点云对象
rospy.loginfo("Converting point cloud message to open3d point cloud...")
pointcloud = o3d.geometry.PointCloud()
pointcloud.points = o3d.utility.Vector3dVector(o3d.io.read_point_cloud_from_msg(pointcloud_msg).points)
# 将点云进行三角化
rospy.loginfo("Triangulating point cloud...")
pointcloud.estimate_normals()
pointcloud.orient_normals_towards_camera_location()
pointcloud.triangulate()
# 获取点云的位姿
rospy.loginfo("Waiting for point cloud transform from {} to world...".format(pointcloud_frame))
transform = rospy.wait_for_message("/tf", tf2_msgs.msg.TFMessage)
transform_stamped = None
for t in transform.transforms:
if t.header.frame_id == pointcloud_frame and t.child_frame_id == "world":
transform_stamped = t
break
if transform_stamped is None:
rospy.logerr("Could not find transform from {} to world".format(pointcloud_frame))
return
pose = PoseStamped()
pose.header.stamp = transform_stamped.header.stamp
pose.header.frame_id = pointcloud_frame
pose.pose.orientation = transform_stamped.transform.rotation
pose.pose.position.x = transform_stamped.transform.translation.x
pose.pose.position.y = transform_stamped.transform.translation.y
pose.pose.position.z = transform_stamped.transform.translation.z
# 将点云位姿转换到 PlanningScene 中的坐标系
rospy.loginfo("Transforming point cloud pose to planning scene frame...")
pose_transformed = do_transform_pose(pose, scene_publisher.frame_id)
# 将点云位姿和颜色添加到 PlanningScene 中
rospy.loginfo("Adding point cloud to planning scene...")
scene_msg = PlanningScene()
scene_msg.is_diff = True
object_msg = moveit_msgs.msg.CollisionObject()
object_msg.id = pointcloud_topic
object_msg.header.frame_id = scene_publisher.frame_id
object_msg.meshes = [o3d.io.read_triangle_mesh_from_point_cloud(pointcloud).create_mesh_primitive()]
object_msg.mesh_poses = [pose_transformed.pose]
object_msg.operation = moveit_msgs.msg.CollisionObject.ADD
object_color = ObjectColor()
object_color.id = pointcloud_topic
object_color.color.r = color[0]
object_color.color.g = color[1]
object_color.color.b = color[2]
object_color.color.a = 1.0
scene_msg.world.collision_objects = [object_msg]
scene_msg.object_colors = [object_color]
scene_publisher.publish(scene_msg)
rospy.loginfo("Done.")
```
这个函数可以将指定 topic 的点云转换成场景中的物体,并发布到 PlanningScene 中。其中,点云会进行 open3d 的三角化处理。您需要传递点云的 topic、点云的坐标系、PlanningScene 的发布者以及物体的颜色等参数。函数会等待点云和位姿的消息,并将它们转换成 PlanningScene 中的坐标系。最后,函数会将点云位姿和颜色添加到 PlanningScene 中。
希望这个函数能够满足您的需求。如有疑问,欢迎继续提问。
阅读全文
相关推荐
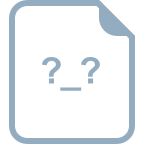











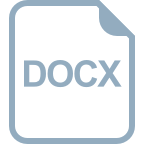
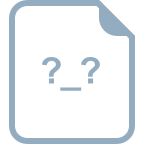
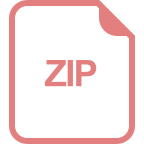
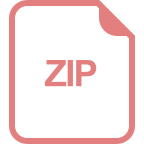
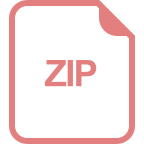