rs485通信软件简单实现
时间: 2023-08-23 16:05:43 浏览: 171
RS485通信是一种常用的串行通信协议,可以用于实现远距离、高速率、多节点的数据传输。在实现RS485通信时,需要使用一个控制器(如单片机)来控制数据的发送和接收,并且需要编写相应的通信协议和通信软件。
下面是一个简单的RS485通信软件实现的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#include <string.h>
#define DEVICE "/dev/ttyUSB0" // 串口设备文件
#define BAUDRATE B9600 // 波特率
#define BUF_SIZE 1024 // 数据缓冲区大小
int main()
{
int fd; // 文件描述符
char buf[BUF_SIZE]; // 数据缓冲区
struct termios opt; // 串口配置结构体
// 打开串口设备
fd = open(DEVICE, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
printf("Open device failed!\n");
return -1;
}
// 配置串口属性
tcgetattr(fd, &opt);
cfsetispeed(&opt, BAUDRATE);
cfsetospeed(&opt, BAUDRATE);
opt.c_cflag |= CLOCAL | CREAD;
opt.c_cflag &= ~CSIZE;
opt.c_cflag |= CS8;
opt.c_cflag &= ~PARENB;
opt.c_cflag &= ~CSTOPB;
opt.c_oflag &= ~OPOST;
opt.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
opt.c_cc[VTIME] = 0;
opt.c_cc[VMIN] = 1;
tcsetattr(fd, TCSANOW, &opt);
// 读取数据
while (1) {
memset(buf, 0, BUF_SIZE);
int n = read(fd, buf, BUF_SIZE);
if (n < 0) {
printf("Read data failed!\n");
break;
} else if (n == 0) {
usleep(1000);
} else {
printf("Receive data: %s\n", buf);
}
}
// 关闭串口设备
close(fd);
return 0;
}
```
这段代码中,首先定义了一个串口设备文件、波特率和数据缓冲区大小。然后打开串口设备,并且使用tcgetattr()和tcsetattr()函数配置串口属性,包括波特率、数据位、停止位等参数。最后使用read()函数读取串口接收到的数据,并且输出到终端中。在实际的应用中,还需要根据通信协议对数据进行解析和处理。
相关推荐
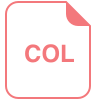













