ubuntu实现RS485通信用C语言
时间: 2024-04-05 12:10:36 浏览: 17
要在Ubuntu下实现RS485通信,你需要使用Linux提供的串口编程接口。以下是一个简单的C语言程序示例,用于通过RS485发送和接收数据:
```c
#include <stdio.h>
#include <string.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
int main()
{
int fd = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("open");
return -1;
}
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag |= (CLOCAL | CREAD);
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_iflag &= ~(IXON | IXOFF | IXANY);
options.c_oflag &= ~OPOST;
options.c_cc[VMIN] = 0;
options.c_cc[VTIME] = 10;
tcsetattr(fd, TCSANOW, &options);
char buffer[256];
memset(buffer, 0, sizeof(buffer));
// 发送数据
strcpy(buffer, "hello");
int len = strlen(buffer);
write(fd, buffer, len);
// 接收数据
len = read(fd, buffer, sizeof(buffer));
if (len > 0) {
printf("received: %s\n", buffer);
}
close(fd);
return 0;
}
```
在这个示例程序中,我们使用串口设备文件`/dev/ttyUSB0`进行通信,你需要将其替换为你使用的串口设备文件。在`options`结构体中,我们设置了波特率为9600,数据位为8位,无奇偶校验,无停止位。我们还关闭了串口的软件流控制和回显,设置了读取超时时间为10秒。在发送数据时,我们将字符串"hello"复制到缓冲区`buffer`中,并使用`write`函数将其发送出去。在接收数据时,我们使用`read`函数从串口中读取数据到缓冲区`buffer`中,并打印出来。
当然,你需要根据你实际的需求进行修改和完善。
相关推荐
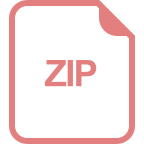
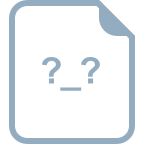
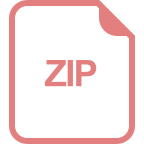














