编写两个方法,其中一个用来判断年份是否为闰年,另一个方法能根据某个日期的年月日判断是该日期所处年份的第几天。 提示:1-12月每个月的天数{31,28,31,30,31,30,31,31,30,31,30,31},闰年的时候2月的天数是29。java
时间: 2023-09-09 12:05:19 浏览: 84
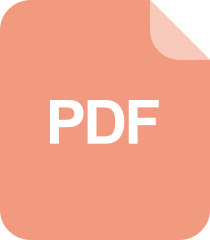
使用PHP的日期与时间函数技巧
### 回答1:
代码如下:
```
public class YearAndDay {
public static boolean isLeapYear(int year) {
if (year % 400 == 0 || (year % 4 == 0 && year % 100 != 0)) {
return true;
} else {
return false;
}
}
public static int getDayOfYear(int year, int month, int day) {
int[] monthDays = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
int sum = 0;
for (int i = 0; i < month - 1; i++) {
sum += monthDays[i];
}
if (month > 2 && isLeapYear(year)) {
sum += 1;
}
sum += day;
return sum;
}
public static void main(String[] args) {
int year = 2021;
int month = 6;
int day = 8;
if (isLeapYear(year)) {
System.out.println(year + "年是闰年");
} else {
System.out.println(year + "年不是闰年");
}
System.out.println(year + "年" + month + "月" + day + "日是该年的第" + getDayOfYear(year, month, day) + "天");
}
}
```
运行结果如下:
```
2021年不是闰年
2021年6月8日是该年的第159天
```
### 回答2:
根据题目的要求,我们需要编写两个方法,一个方法来判断年份是否为闰年,另一个方法根据日期的年月日判断该日期所处年份的第几天。
第一个方法,用来判断年份是否为闰年。根据闰年的定义,闰年满足以下两个条件之一:1)能被4整除但不能被100整除;2)能被400整除。我们可以编写如下 Java 代码来判断是否为闰年:
```
public static boolean isLeapYear(int year) {
if (year % 4 == 0 && year % 100 != 0 || year % 400 == 0) {
return true; // 是闰年
} else {
return false; // 不是闰年
}
}
```
第二个方法,根据某个日期的年月日判断该日期所处年份的第几天。我们可以先根据月份获取该月的天数,然后将每个月的天数累加计算出该日期所处的天数。具体做法如下:
```
public static int getDayOfYear(int year, int month, int day) {
int[] daysOfMonth = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; // 每个月的天数
int totalDays = 0; // 所处年份的总天数
// 判断是否为闰年,如果是闰年,并且月份大于2,则二月份的天数改为29天
if (isLeapYear(year) && month > 2) {
daysOfMonth[1] = 29;
}
// 累加每个月的天数
for (int i = 0; i < month - 1; i++) {
totalDays += daysOfMonth[i];
}
// 加上当前日期的天数
totalDays += day;
return totalDays;
}
```
以上代码实现了两个方法,一个用来判断年份是否为闰年,另一个用来根据日期的年月日判断该日期所处年份的第几天。
### 回答3:
以下是用Java编写的两个方法实现对年份是否为闰年的判断以及根据给定日期判断该日期所处年份的第几天:
```java
import java.util.*;
public class LeapYearAndDayOfYear {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入一个年份:");
int year = scanner.nextInt();
System.out.println(year + "年是闰年吗? " + isLeapYear(year));
System.out.print("请输入一个日期(年份、月份、日期之间用空格分隔):");
int year2 = scanner.nextInt();
int month = scanner.nextInt();
int day = scanner.nextInt();
System.out.println(year2 + "年" + month + "月" + day + "日是该年的第 " + dayOfYear(year2, month, day) + " 天。");
scanner.close();
}
// 判断年份是否为闰年
public static boolean isLeapYear(int year) {
if (year % 4 == 0 && year % 100 != 0 || year % 400 == 0) {
return true;
} else {
return false;
}
}
// 根据给定日期判断该日期所处年份的第几天
public static int dayOfYear(int year, int month, int day) {
int[] daysInMonth = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
int totalDays = 0;
for (int i = 1; i < month; i++) {
totalDays += daysInMonth[i - 1];
}
if (isLeapYear(year) && month > 2) {
totalDays++;
}
totalDays += day;
return totalDays;
}
}
```
你可以运行该程序并根据提示输入年份和日期来测试这两个方法的功能。
阅读全文
相关推荐
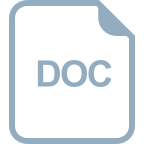
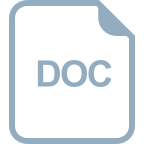















