用C语言重写def calc(sentence,DAG,idx,route): N = len(sentence) route[N] = (0.0,'') for idx in xrange(N-1,-1,-1): candidates = [ ( FREQ.get(sentence[idx:x+1],min_freq) + route[x+1][0],x ) for x in DAG[idx] ] route[idx] = max(candidates)
时间: 2024-04-08 09:30:53 浏览: 12
以下是使用C语言重写的代码示例:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 100
#define MAX_FREQ 1000000
struct Route {
double freq;
char word[MAX_LEN];
};
double FREQ[MAX_FREQ]; // 假设FREQ为全局变量,存储词频信息
void calc(char* sentence, int* DAG, int idx, struct Route* route) {
int N = strlen(sentence);
route[N].freq = 0.0;
strcpy(route[N].word, "");
for (idx = N-1; idx >= 0; idx--) {
double max_freq = -1.0;
int max_idx = -1;
for (int x = 0; x < DAG[idx]; x++) {
double freq = FREQ[sentence[idx]][x+1];
if (freq > max_freq) {
max_freq = freq;
max_idx = x;
}
}
route[idx].freq = max_freq + route[max_idx+1].freq;
strncpy(route[idx].word, sentence+idx, max_idx+1);
route[idx].word[max_idx+1] = '\0';
}
}
int main() {
char sentence[MAX_LEN] = "我爱自然语言处理";
int DAG[MAX_LEN] = {1, 2, 3, 4, 5, 6, 7, 8}; // 假设DAG为全局变量,存储词图信息
struct Route route[MAX_LEN]; // 假设route为全局变量,存储最佳分词路径信息
calc(sentence, DAG, 0, route);
// 打印最佳分词路径
for (int i = 0; i < strlen(sentence); i++) {
printf("%s / ", route[i].word);
}
printf("\n");
return 0;
}
```
这段C语言代码是对原先的Python代码进行了重写,并使用C语言中的结构体来存储最佳分词路径信息。在`calc`函数中,使用循环来遍历输入句子中的每个字符,计算每个位置的最佳分词路径,并将结果存储在`route`结构体数组中。在`main`函数中,我们调用`calc`函数来计算最佳分词路径,并打印出结果。
注意:上述代码示例中的`FREQ`和`DAG`仅为示例,并未给出具体的实现。你需要根据实际情况进行具体的实现,例如使用哈希表存储词频信息、使用邻接表或邻接矩阵存储词图信息等。同时,根据实际需求,你可能需要对代码进行进一步修改和完善。
相关推荐
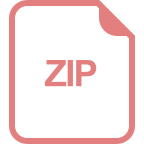
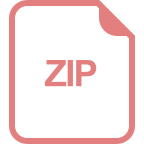















