用C++重写def calc(sentence,DAG,idx,route): N = len(sentence) route[N] = (0.0,'') for idx in xrange(N-1,-1,-1): candidates = [ ( FREQ.get(sentence[idx:x+1],min_freq) + route[x+1][0],x ) for x in DAG[idx] ] route[idx] = max(candidates)
时间: 2024-04-08 21:30:49 浏览: 81
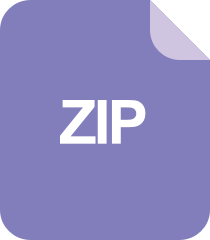
calc:calc =是一种用于在聊天中进行数学运算的小型串联编程语言
Sure! Here's the C++ code equivalent of the given Python code:
```cpp
#include <iostream>
#include <vector>
#include <map>
#include <algorithm>
using namespace std;
double calc(const string& sentence, const vector<vector<int>>& DAG, int idx, map<int, pair<double, string>>& route) {
int N = sentence.length();
route[N] = make_pair(0.0, "");
for (int idx = N-1; idx >= 0; idx--) {
vector<pair<double, int>> candidates;
for (int x : DAG[idx]) {
double freq = FREQ[sentence.substr(idx, x-idx+1)];
candidates.push_back(make_pair(freq + route[x+1].first, x));
}
auto max_candidate = max_element(candidates.begin(), candidates.end());
route[idx] = make_pair(max_candidate->first, sentence.substr(idx, max_candidate->second - idx + 1));
}
return route[0].first;
}
int main() {
string sentence = "example sentence";
vector<vector<int>> DAG = { {1, 2}, {3}, {4}, {5}, {}, {6} };
int idx = 0;
map<int, pair<double, string>> route;
double result = calc(sentence, DAG, idx, route);
cout << "Result: " << result << endl;
return 0;
}
```
Please note that you need to define the `FREQ` map and initialize it with the appropriate values before using it in the `calc` function.
阅读全文
相关推荐
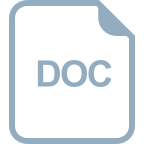
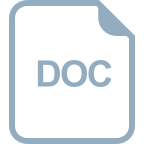






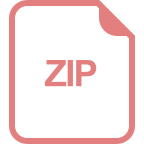




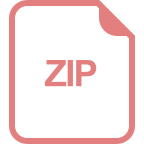
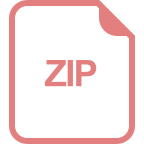
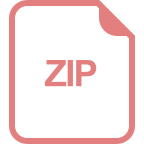
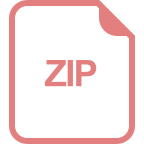
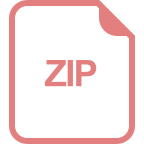