利用python编写一段代码,要求完成三线程顺序打印出正整数,从1开始打印到100,其中a线程打印3的倍数,b线程打印5的倍数,c线程打印其他数字
时间: 2024-02-28 09:55:09 浏览: 83
好的,以下是符合您要求的Python代码:
```python
import threading
class PrintThread(threading.Thread):
def __init__(self, start, end, step, lock, condition):
super().__init__()
self.start = start
self.end = end
self.step = step
self.lock = lock
self.condition = condition
def run(self):
for i in range(self.start, self.end + 1, self.step):
with self.lock:
self.condition.wait()
print(i, end=" ")
self.condition.notify_all()
if __name__ == "__main__":
lock = threading.Lock()
condition_a = threading.Condition(lock)
condition_b = threading.Condition(lock)
condition_c = threading.Condition(lock)
thread_a = PrintThread(3, 100, 3, lock, condition_a)
thread_b = PrintThread(5, 100, 5, lock, condition_b)
thread_c = PrintThread(1, 100, 1, lock, condition_c)
thread_a.start()
thread_b.start()
thread_c.start()
with lock:
condition_a.notify_all()
thread_a.join()
thread_b.join()
thread_c.join()
```
代码中创建了三个不同的线程,每个线程负责打印不同的数字。这三个线程共享一个锁和三个条件变量,以确保它们的打印顺序正确。
线程a打印3的倍数,在构造函数中传入了起始点3,步长3,以及一个条件变量condition_a。线程b打印5的倍数,在构造函数中传入了起始点5,步长5,以及一个条件变量condition_b。线程c打印其他数字,在构造函数中传入了起始点1,步长1,以及一个条件变量condition_c。
在每个线程的run方法中,使用了with语句锁住了共享锁。然后使用条件变量的wait()方法等待通知。在打印完数字后,使用notify_all()方法通知其他线程可以执行。最后,在主线程中通知线程a开始执行,并等待三个线程全部执行完毕。
阅读全文
相关推荐
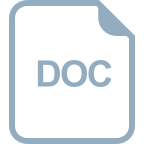
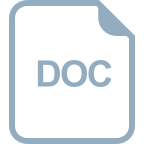
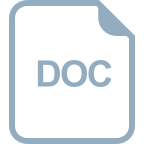
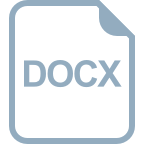
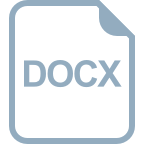
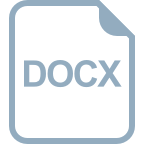
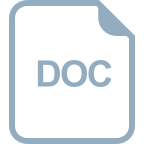
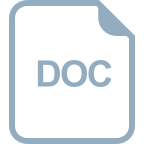
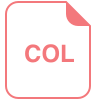
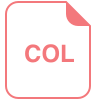
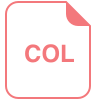
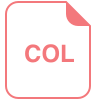
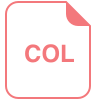





