python 遍历文件内所有名称含result的文件,并计数数量
时间: 2024-10-07 17:06:43 浏览: 22
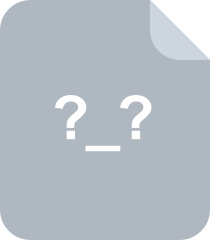
python3实现通过文件后缀的筛选文件
在Python中,你可以使用`os`模块的`listdir()`函数配合递归来遍历文件夹内的所有文件,并使用正则表达式检查文件名是否包含"result"。以下是如何实现这个功能的一个示例:
```python
import os
def count_files_with_result(directory):
file_count = 0
for root, dirs, files in os.walk(directory): # 使用os.walk遍历目录及其子目录
for file in files:
if "result" in file: # 检查文件名是否包含 "result"
file_count += 1
print(f"File found: {file}, Directory: {root}")
return file_count
# 调用函数并传入要搜索的根目录
directory_to_search = "./your_directory_path" # 替换为你实际的目录路径
count = count_files_with_result(directory_to_search)
print(f"Total number of files with 'result': {count}")
阅读全文
相关推荐
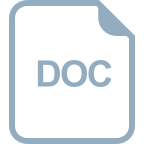
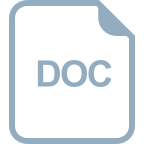
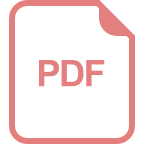
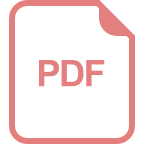
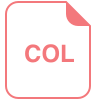
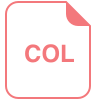
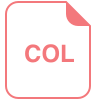
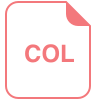









