对已建立的顺序表实现插入,删除等基本操作,写出C语言代码
时间: 2024-09-14 12:08:50 浏览: 51
在C语言中,我们可以使用结构体和指针来实现顺序表的基本操作,如插入和删除。这里是一个简单的顺序表实现示例,我们将创建一个`ListNode`结构体作为元素,并提供`Insert`, `Delete`, 和 `Search` 函数:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义顺序表节点
typedef struct ListNode {
int data;
struct ListNode* next;
} ListNode;
// 插入节点到链表头部
void Insert(ListNode** head, int value) {
// 分配新的节点内存
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
// 设置新节点数据并将其设为首节点
newNode->data = value;
newNode->next = *head;
// 更新头节点指向新节点
*head = newNode;
}
// 删除指定值的节点(从头开始搜索)
void Delete(ListNode** head, int value) {
if (*head == NULL) {
printf("List is empty.\n");
return;
}
// 遍历查找目标节点
ListNode* current = *head;
while (current != NULL && current->data != value) {
current = current->next;
}
// 如果找到节点,更新前一个节点的next指针
if (current != NULL) {
ListNode* temp = current->next;
free(current); // 释放已删除节点的内存
*head = temp; // 将当前节点替换为下一个节点
} else {
printf("Value not found in the list.\n");
}
}
// 查找特定值的节点,返回第一个匹配项的地址,如果没有则返回NULL
ListNode* Search(ListNode* head, int value) {
ListNode* current = head;
while (current != NULL) {
if (current->data == value) {
return current;
}
current = current->next;
}
return NULL;
}
int main() {
// 示例演示
ListNode* head = NULL;
Insert(&head, 5);
Insert(&head, 10);
Insert(&head, 15);
printf("Before deletion: ");
ListNode* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
Delete(&head, 10);
printf("After deleting 10: ");
current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
return 0;
}
阅读全文
相关推荐
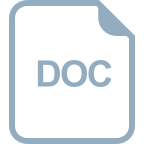
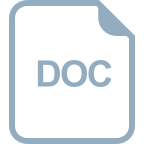
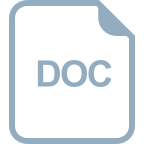















