已知一个长度为6多有序顺序表 2 ,6 ,7 ,10, 12 18,设计一个算法插入一个值为8的节点 使其插入后的顺序表仍然有序。用c语言写出完整代码
时间: 2024-09-29 10:10:53 浏览: 31
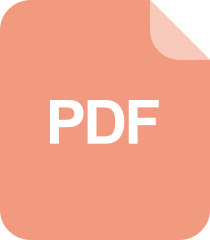
江南大学《数据结构与算法A》2022-2023学年第一学期期末试卷A(带答案)
为了在有序顺序表中插入一个新值并保持其有序,我们可以遍历列表,找到合适的位置将8插入。这里是一个简单的C语言算法描述以及示例代码:
1. 创建一个函数,接收两个参数:有序顺序表的头指针`head`和要插入的新值`value`。
2. 遍历数组,如果当前元素小于新值,则继续移动到下一个元素,直到找到大于等于新值的位置。
3. 插入新值到找到的位置,并调整相邻元素的指针。
以下是C语言代码实现:
```c
#include <stdio.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
// 插入新值并保持有序
Node* insertInOrder(Node* head, int value) {
if (head == NULL || head->data >= value) {
// 如果链表为空或新值应在头部,直接添加
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = head;
return newNode;
}
Node* current = head;
while (current->next != NULL && current->next->data < value) {
current = current->next;
}
// 在找到适当位置后插入新节点
newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = current->next;
current->next = newNode;
return head;
}
// 示例:创建有序顺序表并插入8
int main() {
Node* list = NULL; // 初始化空链表
// 添加初始元素
list = insertInOrder(list, 2);
list = insertInOrder(list, 6);
list = insertInOrder(list, 7);
list = insertInOrder(list, 10);
list = insertInOrder(list, 12);
list = insertInOrder(list, 18);
// 插入8
list = insertInOrder(list, 8);
// 打印排序后的顺序表
Node* temp = list;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
// 释放内存
free(temp); // 只能打印最后一位节点,因为递归终止会在临时变量上
return 0;
}
```
运行上述代码后,你会看到插入8后的有序顺序表:2 -> 6 -> 7 -> 8 -> 10 -> 12 -> 18 -> NULL。
阅读全文
相关推荐
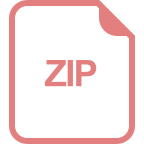
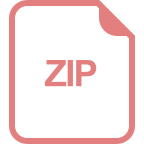


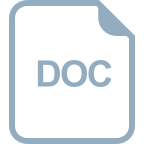












