用C语言代码,解决分治法求距离最近点对问题
时间: 2024-10-12 10:04:53 浏览: 37
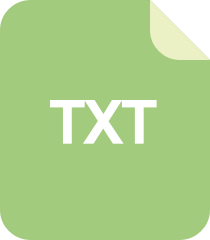
递归的解决最近问题
分治法求解距离最近点对问题,通常涉及排序和比较操作,最经典的算法之一是Floyd-Warshall算法。但是,如果你想要的是在线性时间复杂度下寻找单对最短路径,可以考虑使用“两个指针”技巧,假设输入是一个二维数组表示两点之间的边长。以下是简化版的C语言代码示例,用于在一个二维数组上找到距离最短的点对:
```c
#include <stdio.h>
// 定义结构体存储点的坐标和值
typedef struct {
int x, y; // 点的坐标
int val; // 点的值(即到原点的距离)
} Point;
// 比较函数,用于排序
int compare(const void* a, const void* b) {
return ((Point*)a)->val - ((Point*)b)->val;
}
// 寻找距离最近的点对
void findClosestPair(int arr[][2], int n) {
Point points[n];
for (int i = 0; i < n; i++) {
points[i].x = arr[i][0];
points[i].y = arr[i][1];
points[i].val = sqrt(points[i].x * points[i].x + points[i].y * points[i].y); // 到原点的距离
}
qsort(points, n, sizeof(Point), compare);
int best_dist = INT_MAX, closest_pair[2] = {0, 0};
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (points[j].val - points[i].val < best_dist) {
best_dist = points[j].val - points[i].val;
closest_pair[0] = i;
closest_pair[1] = j;
}
}
}
printf("Distance between the closest pair is: %d\n", best_dist);
printf("(%d, %d) and (%d, %d)\n", points[closest_pair[0]].x, points[closest_pair[0]].y,
points[closest_pair[1]].x, points[closest_pair[1]].y);
}
int main() {
int arr[][] = {{0, 0}, {2, 3}, {5, 4}};
int n = sizeof(arr) / sizeof(arr[0]);
findClosestPair(arr, n);
return 0;
}
```
这个程序首先计算每个点到原点的距离并按距离降序排列。然后遍历所有组合,找出最小差值对应的点对。
阅读全文
相关推荐
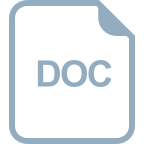
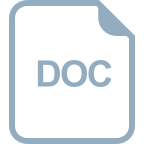



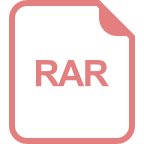










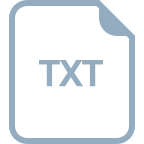
