给定 N 给出一个单链表,不知道节点N的值,怎样只遍历一次就可以求出中间结点。输入样例:3 -> 4 -> 7 -> 9 ->12 ->5 ->8
时间: 2024-06-06 20:06:52 浏览: 8
解题思路:
快慢指针法,即使用两个指针,一个指针每次走两步,一个指针每次走一步,当快指针走到链表末尾时,慢指针就到了链表的中间。
算法步骤:
1.定义快慢指针,初始化为head节点。
2.循环遍历链表,快指针每次走两步,慢指针每次走一步。
3.当快指针走到链表末尾时,慢指针就到了链表的中间。
4.返回慢指针指向的节点即可。
Python代码实现:
相关问题
假定一棵二叉树一个结点用一个字符描述,现在给出中序和按层遍历的字符串,求该树 的先序遍历字符串。 样例输入 DBEAC ABCDE 样例输出 ABDEC 用c++实现
题目描述
给定二叉树的中序遍历序列和按层遍历序列,求该二叉树的先序遍历序列。
样例输入
DBEAC ABCDE
样例输出
ABDEC
算法思路
该题解法与上一题类似,我们可以通过中序遍历和按层遍历重构出二叉树,然后再进行先序遍历即可。
具体步骤如下:
- 构建一个无序哈希表,用于记录中序遍历中每个字符的位置,以便于后面快速确定左右子树。
- 以按层遍历的第一个字符作为根节点,创建一个队列,将根节点入队。
- 遍历按层遍历序列,对于每个字符,都创建一个新的节点,并根据中序遍历序列的位置,将其放置在相应的位置上。
- 如果当前遍历到的字符在队首节点的左子树中,就将其作为队首节点的左子节点,否则就将其作为队首节点的右子节点。如果一个节点有右子节点,那么它将会被弹出队列,直到找到它的父节点。
代码实现
以下是用C++实现的代码:
用C语言写出要求按照先序遍历的顺序输出给定二叉树的叶结点,并且你给出一个二叉树代入程序中
以下是用C语言实现先序遍历输出二叉树叶子节点的代码,假设二叉树的叶子节点存储为0:
```c
#include <stdio.h>
#include <stdlib.h>
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
void preorder(struct TreeNode *root) {
if (root == NULL) {
return;
}
if (root->left == NULL && root->right == NULL) {
printf("%d ", root->val);
}
preorder(root->left);
preorder(root->right);
}
int main() {
struct TreeNode *root = (struct TreeNode *)malloc(sizeof(struct TreeNode));
root->val = 1;
root->left = (struct TreeNode *)malloc(sizeof(struct TreeNode));
root->left->val = 2;
root->left->left = (struct TreeNode *)malloc(sizeof(struct TreeNode));
root->left->left->val = 0;
root->left->right = (struct TreeNode *)malloc(sizeof(struct TreeNode));
root->left->right->val = 0;
root->right = (struct TreeNode *)malloc(sizeof(struct TreeNode));
root->right->val = 3;
root->right->left = (struct TreeNode *)malloc(sizeof(struct TreeNode));
root->right->left->val = 4;
root->right->right = (struct TreeNode *)malloc(sizeof(struct TreeNode));
root->right->right->val = 5;
root->right->right->left = (struct TreeNode *)malloc(sizeof(struct TreeNode));
root->right->right->left->val = 0;
root->right->right->right = (struct TreeNode *)malloc(sizeof(struct TreeNode));
root->right->right->right->val = 0;
preorder(root);
return 0;
}
```
上述代码中先定义了一个二叉树的结构体,包含节点的值和左右子节点的指针,然后通过先序遍历的方式遍历整个二叉树,当遇到叶子节点时输出其值即可。最后在main函数中创建了一个二叉树并调用preorder函数进行遍历输出。
相关推荐
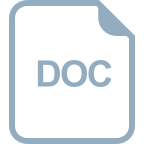
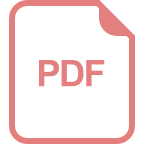












