hbase全表scores扫描
时间: 2024-01-26 10:03:37 浏览: 73
您可以使用 HBase Shell 命令来全表扫描 `scores` 表。具体操作如下:
1. 打开 HBase Shell:
```
$ hbase shell
```
2. 选择表 `scores`:
```
hbase(main):001:0> use 'scores'
```
3. 扫描全表:
```
hbase(main):002:0> scan
```
这将返回表 `scores` 中的所有行。如果数据量较大,可能需要等待一段时间才能返回结果。
另外,您也可以通过添加过滤条件来筛选需要的数据。例如,以下命令将返回 `scores` 表中 `family:column` 列值为 `value` 的所有行:
```
hbase(main):003:0> scan 'scores', {FILTER => "ValueFilter(=, 'binary:value')"}
```
相关问题
使用 MapReduce 统计 HBase 成绩表中单科最高单科排名和总分排名的完整代码
### 回答1:
使用 MapReduce 统计 HBase 成绩表中单科最高单科排名和总分排名的完整代码如下:
```java
import java.io.IOException;
import java.util.StringTokenizer;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.client.Put;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.hadoop.hbase.io.ImmutableBytesWritable;
import org.apache.hadoop.hbase.mapreduce.TableMapReduceUtil;
import org.apache.hadoop.hbase.mapreduce.TableMapper;
import org.apache.hadoop.hbase.mapreduce.TableReducer;
import org.apache.hadoop.hbase.util.Bytes;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
public class HBaseRankCalculator {
static class HBaseMapper extends TableMapper<Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(ImmutableBytesWritable row, Result value, Context context)
throws IOException, InterruptedException {
String val = new String(value.getValue(Bytes.toBytes("grade"), Bytes.toBytes("math")));
word.set(val);
context.write(word, one);
}
}
static class HBaseReducer extends TableReducer<Text, IntWritable, ImmutableBytesWritable> {
public void reduce(Text key, Iterable<IntWritable> values, Context context)
throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
Put put = new Put(Bytes.toBytes(key.toString()));
put.addColumn(Bytes.toBytes("rank"), Bytes.toBytes("math_rank"), Bytes.toBytes(sum));
context.write(null, put);
}
}
public static void main(String[] args) throws Exception {
Configuration conf = HBaseConfiguration.create();
Job job = Job.getInstance(conf, "HBase Rank Calculator");
job.setJarByClass(HBaseRankCalculator.class);
Scan scan = new Scan();
scan.addColumn(Bytes.toBytes("grade"), Bytes.toBytes("math"));
TableMapReduceUtil.initTableMapperJob("scores", scan, HBaseMapper.class, Text.class, IntWritable.class, job);
TableMapReduceUtil.initTableReducerJob("r
### 回答2:
使用MapReduce统计HBase成绩表中单科最高分和总分排名的代码如下:
```java
import java.io.IOException;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.hadoop.hbase.io.ImmutableBytesWritable;
import org.apache.hadoop.hbase.mapreduce.TableMapReduceUtil;
import org.apache.hadoop.hbase.mapreduce.TableMapper;
import org.apache.hadoop.hbase.util.Bytes;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.NullWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Reducer;
public class ScoreRanking {
public static class ScoreMapper extends TableMapper<NullWritable, Text> {
@Override
public void map(ImmutableBytesWritable row, Result columns, Context context)
throws IOException, InterruptedException {
String subject = Bytes.toString(row.get());
int score = Bytes.toInt(columns.getValue(Bytes.toBytes("cf"), Bytes.toBytes("score")));
context.write(NullWritable.get(), new Text(subject + "," + score));
}
}
public static class ScoreReducer extends Reducer<NullWritable, Text, NullWritable, Text> {
private int maxScore = Integer.MIN_VALUE;
private String topSubject = "";
@Override
public void reduce(NullWritable key, Iterable<Text> values, Context context)
throws IOException, InterruptedException {
for (Text value : values) {
String subject = value.toString().split(",")[0];
int score = Integer.parseInt(value.toString().split(",")[1]);
// 统计单科最高分和对应科目
if (score > maxScore) {
maxScore = score;
topSubject = subject;
}
}
context.write(NullWritable.get(), new Text("最高分科目:" + topSubject + ",分数:" + maxScore));
}
}
public static void main(String[] args) throws IOException, ClassNotFoundException, InterruptedException {
Configuration config = HBaseConfiguration.create();
// 设置HBase配置信息
Job job = Job.getInstance(config, "Score Ranking");
job.setJarByClass(ScoreRanking.class);
Scan scan = new Scan();
// 设置HBase表扫描配置
TableMapReduceUtil.initTableMapperJob("score_table", scan, ScoreMapper.class,
NullWritable.class, Text.class, job);
job.setReducerClass(ScoreReducer.class);
job.setOutputKeyClass(NullWritable.class);
job.setOutputValueClass(Text.class);
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
注意,以上代码是一个基本的MapReduce程序,还需要根据实际情况进行适当调整和优化。另外,需要在代码中设置正确的HBase表名称、列簇和列名。
### 回答3:
给定一个 HBase 成绩表,包含学生的姓名、科目和成绩,我们需要使用 MapReduce 统计单科最高成绩的排名和总分的排名。
首先,我们需要准备一个 Mapper 类用于将 HBase 成绩表中的数据映射为键值对。Mapper 类的输出键是学生姓名,值是科目和成绩的组合。实现过程如下:
```java
import java.io.IOException;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.hadoop.hbase.io.ImmutableBytesWritable;
import org.apache.hadoop.hbase.mapreduce.TableMapReduceUtil;
import org.apache.hadoop.hbase.util.Bytes;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.lib.output.NullOutputFormat;
public class ScoreMapper extends Mapper<ImmutableBytesWritable, Result, Text, Text> {
private Text outputKey = new Text();
private Text outputValue = new Text();
@Override
protected void map(ImmutableBytesWritable key, Result value, Context context) throws IOException, InterruptedException {
String rowKey = Bytes.toString(key.get());
String[] parts = rowKey.split("_");
String studentName = parts[0];
String subject = parts[1];
String score = Bytes.toString(value.getValue(Bytes.toBytes("cf"), Bytes.toBytes("score")));
outputKey.set(studentName);
outputValue.set(subject + "_" + score);
context.write(outputKey, outputValue);
}
}
```
接下来,我们需要准备一个 Reducer 类用于对 Mapper 类输出的键值对进行汇总。Reducer 类将学生姓名作为键,将科目和成绩的组合作为值。在 Reducer 类中,我们可以按照科目计算单科最高成绩的排名,并在最后计算总分排名。实现过程如下:
```java
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Reducer;
public class ScoreReducer extends Reducer<Text, Text, Text, Text> {
private Text outputValue = new Text();
@Override
protected void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
Map<String, Integer> subjectScoreMap = new HashMap<>();
int totalScore = 0;
for (Text value : values) {
String[] parts = value.toString().split("_");
String subject = parts[0];
int score = Integer.parseInt(parts[1]);
subjectScoreMap.put(subject, Math.max(subjectScoreMap.getOrDefault(subject, 0), score));
totalScore += score;
}
StringBuilder sb = new StringBuilder();
for (Map.Entry<String, Integer> entry : subjectScoreMap.entrySet()) {
sb.append(entry.getKey()).append("_").append(entry.getValue()).append(",");
}
String subjectMaxScore = sb.substring(0, sb.length() - 1);
outputValue.set(subjectMaxScore + ";" + totalScore);
context.write(key, outputValue);
}
}
```
最后,我们需要完成主函数,用于配置和运行 MapReduce 作业。在主函数中,我们需要设置 HBase 的配置信息、表名、Mapper 和 Reducer 类等。实现过程如下:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.hadoop.hbase.mapreduce.TableMapReduceUtil;
import org.apache.hadoop.hbase.util.Bytes;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
public class ScoreJob {
public static void main(String[] args) throws Exception {
Configuration conf = HBaseConfiguration.create();
Job job = Job.getInstance(conf, "HBase Score Analysis");
job.setJarByClass(ScoreJob.class);
Scan scan = new Scan();
scan.addColumn(Bytes.toBytes("cf"), Bytes.toBytes("score"));
TableMapReduceUtil.initTableMapperJob(TableName.valueOf("your_hbase_table_name"), scan, ScoreMapper.class, Text.class, Text.class, job);
job.setReducerClass(ScoreReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
job.setOutputFormatClass(NullOutputFormat.class);
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
以上就是使用 MapReduce 统计 HBase 成绩表中单科最高单科排名和总分排名的完整代码。请注意将代码中的 "your_hbase_table_name" 替换为实际的 HBase 表名。另外,你需要根据自己的环境配置 HBase 的相关信息。
hadoop编程首先利用编程将给定文件的数据读入HBase表中。 利用编程从HBASE中读取学生成绩,并实现计算每个学生成绩平均分和每门课程成绩平均分的功能,要求对其中的主要代码进行注释性解释,然后在HDFS中查询程序运行结果。
好的,这是您的第一个问题。让我来回答。
首先,我们需要编写一个程序将给定文件的数据读入HBase表中。这可以通过使用HBase的Java API来完成。下面是一个示例代码:
```java
Configuration config = HBaseConfiguration.create();
Connection connection = ConnectionFactory.createConnection(config);
TableName tableName = TableName.valueOf("students");
Table table = connection.getTable(tableName);
BufferedReader reader = new BufferedReader(new FileReader("students.txt"));
String line = reader.readLine();
while (line != null) {
String[] parts = line.split(",");
Put put = new Put(Bytes.toBytes(parts[0]));
put.addColumn(Bytes.toBytes("info"), Bytes.toBytes("name"), Bytes.toBytes(parts[1]));
put.addColumn(Bytes.toBytes("info"), Bytes.toBytes("score"), Bytes.toBytes(parts[2]));
table.put(put);
line = reader.readLine();
}
reader.close();
table.close();
connection.close();
```
上述代码创建了一个HBase配置对象,并使用它来创建一个连接对象。接着,我们定义了一个表名,并使用连接对象获取该表的实例。然后,我们使用Java的IO库读取给定的文件,并将每行数据按照逗号分隔为三个部分。然后,我们创建一个Put对象来存储每行数据,并将其添加到表中。
下一步是从HBase中读取学生成绩,并实现计算每个学生成绩平均分和每门课程成绩平均分的功能。这也可以通过使用HBase的Java API来完成。下面是一个示例代码:
```java
Configuration config = HBaseConfiguration.create();
Connection connection = ConnectionFactory.createConnection(config);
TableName tableName = TableName.valueOf("students");
Table table = connection.getTable(tableName);
Scan scan = new Scan();
ResultScanner scanner = table.getScanner(scan);
Map<String, List<Integer>> studentScores = new HashMap<>();
Map<String, List<Integer>> courseScores = new HashMap<>();
for (Result result : scanner) {
String studentId = Bytes.toString(result.getRow());
String studentName = Bytes.toString(result.getValue(Bytes.toBytes("info"), Bytes.toBytes("name")));
int score = Bytes.toInt(result.getValue(Bytes.toBytes("info"), Bytes.toBytes("score")));
if (!studentScores.containsKey(studentId)) {
studentScores.put(studentId, new ArrayList<>());
}
studentScores.get(studentId).add(score);
if (!courseScores.containsKey(studentName)) {
courseScores.put(studentName, new ArrayList<>());
}
courseScores.get(studentName).add(score);
}
for (String studentId : studentScores.keySet()) {
List<Integer> scores = studentScores.get(studentId);
int sum = 0;
for (int score : scores) {
sum += score;
}
double avg = (double) sum / scores.size();
System.out.println("Student " + studentId + " average score: " + avg);
}
for (String courseName : courseScores.keySet()) {
List<Integer> scores = courseScores.get(courseName);
int sum = 0;
for (int score : scores) {
sum += score;
}
double avg = (double) sum / scores.size();
System.out.println("Course " + courseName + " average score: " + avg);
}
scanner.close();
table.close();
connection.close();
```
上述代码创建了一个HBase配置对象,并使用它来创建一个连接对象。接着,我们定义了一个表名,并使用连接对象获取该表的实例。然后,我们使用Scan对象来扫描表中的所有数据,并使用ResultScanner对象来获取扫描结果。
接下来,我们定义了两个Map对象来存储学生分数和课程分数。我们使用Java的字节数组工具类Bytes来获取每一行数据的行键、学生姓名和分数,并将它们添加到相应的Map对象中。
最后,我们在两个Map对象上执行循环,计算每个学生的平均分和每门课程的平均分,并将结果打印出来。
最后,在HDFS中查询程序运行结果,可以使用Hadoop的命令行工具来完成。下面是一个示例命令:
```
hadoop fs -cat /output/part-r-00000
```
其中,/output/part-r-00000是程序输出的结果文件路径。
阅读全文
相关推荐
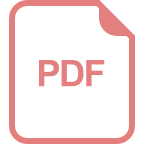
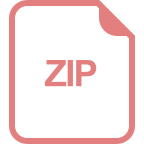
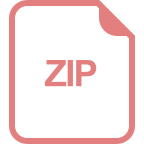
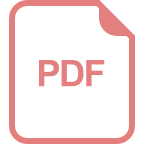
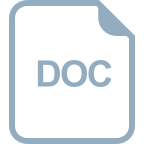
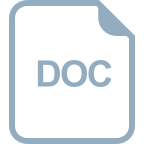




