用一个函数让t的值每秒增加一同时能够运行其他函数,使用c++
时间: 2023-06-27 20:08:14 浏览: 55
可以使用多线程来实现这个功能。以下是一个示例代码:
```c++
#include <iostream>
#include <thread>
#include <chrono>
using namespace std;
void increaseT(int& t) {
while (true) {
this_thread::sleep_for(chrono::seconds(1)); // 每秒钟增加一次
t++;
}
}
void doOtherThings() {
while (true) {
cout << "I am doing other things." << endl;
this_thread::sleep_for(chrono::milliseconds(500)); // 每 0.5 秒进行一次其他操作
}
}
int main() {
int t = 0;
thread t1(increaseT, ref(t)); // 启动一个新的线程来执行 increaseT 函数
thread t2(doOtherThings); // 启动另一个线程来执行 doOtherThings 函数
while (true) {
cout << "t = " << t << endl;
this_thread::sleep_for(chrono::seconds(1)); // 每秒钟输出一次 t 的值
}
return 0;
}
```
在这个示例代码中,我们定义了两个函数 `increaseT` 和 `doOtherThings`,分别用于每秒钟增加变量 `t` 的值和进行其他操作。我们使用 `std::thread` 类来创建两个线程来执行这两个函数。同时,我们在主函数中也通过循环来输出变量 `t` 的值,以便观察 `increaseT` 函数是否能够正常运行。
在 `increaseT` 函数中,我们使用 `std::this_thread::sleep_for` 函数来让线程每秒钟暂停一次,然后将变量 `t` 的值加一。在 `doOtherThings` 函数中,我们也使用了 `std::this_thread::sleep_for` 函数来让线程每 0.5 秒暂停一次,然后输出一些信息。
需要注意的是,在主函数中,我们使用了一个无限循环来输出变量 `t` 的值。这个循环会一直运行,直到程序被终止。因此,我们需要在程序结束的时候手动终止它。
相关推荐
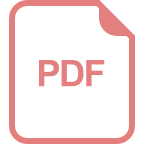
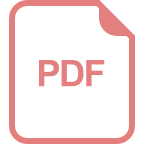














