帮我用C++写一个10*10的扫雷小游戏
时间: 2024-06-09 13:07:04 浏览: 7
好的,以下是一个简单的10*10扫雷游戏的C++代码,仅供参考:
```c++
#include <iostream>
#include <ctime>
using namespace std;
const int SIZE = 10;
const int MINE_NUM = 10;
void init_board(int board[SIZE][SIZE], int mine_num) {
// 初始化棋盘,所有格子都是0,-1表示地雷
for (int i = 0; i < SIZE; ++i) {
for (int j = 0; j < SIZE; ++j) {
board[i][j] = 0;
}
}
// 随机放置地雷
srand(time(nullptr));
int count = 0;
while (count < mine_num) {
int row = rand() % SIZE;
int col = rand() % SIZE;
if (board[row][col] == 0) {
board[row][col] = -1;
++count;
}
}
}
void print_board(int board[SIZE][SIZE], bool show_mines = false) {
// 打印棋盘
cout << " ";
for (int j = 0; j < SIZE; ++j) {
cout << " " << j;
}
cout << endl;
for (int i = 0; i < SIZE; ++i) {
cout << i;
for (int j = 0; j < SIZE; ++j) {
if (board[i][j] >= 0 && !show_mines) {
cout << " .";
} else if (board[i][j] == -1) {
cout << " *";
} else {
cout << " " << board[i][j];
}
}
cout << endl;
}
}
int count_mines(int board[SIZE][SIZE], int row, int col) {
// 统计(row, col)周围的地雷数量
int count = 0;
for (int i = max(0, row - 1); i <= min(SIZE - 1, row + 1); ++i) {
for (int j = max(0, col - 1); j <= min(SIZE - 1, col + 1); ++j) {
if (board[i][j] == -1) {
++count;
}
}
}
return count;
}
void mark_mines(int board[SIZE][SIZE]) {
// 标记所有地雷
for (int i = 0; i < SIZE; ++i) {
for (int j = 0; j < SIZE; ++j) {
if (board[i][j] == -1) {
board[i][j] = -2;
}
}
}
}
bool play_game(int board[SIZE][SIZE]) {
// 主循环,玩家每次输入一个坐标,程序判断是否胜利或失败
int remain = SIZE * SIZE - MINE_NUM;
while (remain > 0) {
print_board(board);
cout << "Please enter row and column: ";
int row, col;
cin >> row >> col;
if (board[row][col] == -1) {
// 点到了地雷,游戏结束
cout << "Boom! You lose!" << endl;
mark_mines(board);
print_board(board, true);
return false;
} else if (board[row][col] > 0) {
// 已经翻开了该格子,重新输入坐标
cout << "This square has been turned over, please select again." << endl;
} else {
// 翻开该格子
int count = count_mines(board, row, col);
board[row][col] = count;
remain--;
if (count == 0) {
// 如果该格子周围没有地雷,则翻开周围的所有格子
for (int i = max(0, row - 1); i <= min(SIZE - 1, row + 1); ++i) {
for (int j = max(0, col - 1); j <= min(SIZE - 1, col + 1); ++j) {
if (board[i][j] == 0) {
count = count_mines(board, i, j);
board[i][j] = count;
remain--;
}
}
}
}
if (remain == 0) {
// 所有非地雷格子都已被翻开,游戏胜利
cout << "Congratulations! You win!" << endl;
mark_mines(board);
print_board(board, true);
return true;
}
}
}
return false;
}
int main() {
int board[SIZE][SIZE];
init_board(board, MINE_NUM);
play_game(board);
return 0;
}
```
这个程序使用了一个二维数组来表示棋盘,每个格子的值表示该格子周围的地雷数量,如果该格子是地雷,则值为-1。玩家每次输入一个坐标,程序判断该格子是否是地雷,如果是,游戏结束;如果不是,则将该格子的值更新为周围的地雷数量,并且翻开周围的所有格子,直到所有非地雷格子都被翻开,游戏胜利。
相关推荐
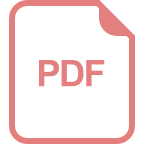
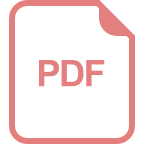









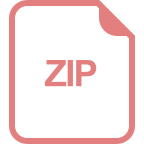
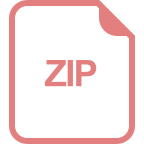
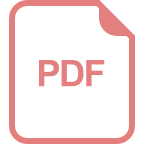
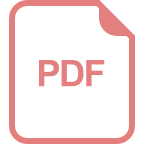
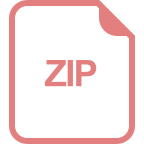