给字符串类定义重载运算函数复制运算符=
时间: 2024-10-16 18:10:07 浏览: 12
在C++中,给字符串类定义重载运算符`=`, 通常是为了实现字符串的赋值操作。当你想要让一个字符串变量复制另一个字符串的内容时,可以重写`=`运算符,使其能够接收另一个字符串作为参数并将其内容复制到目标字符串中。
例如,你可以这样做:
```cpp
class MyString {
private:
std::string data;
public:
// 定义复制构造函数,用于初始化新创建的对象
MyString(const std::string& other) : data(other) {}
// 重载赋值运算符=
MyString& operator=(const std::string& other) {
if (this != &other) { // 防止自我赋值导致无限递归
data = other; // 将其他字符串的内容复制到当前对象的数据成员
}
return *this; // 返回指向自身的引用,允许链式赋值
}
// 其他成员函数...
};
```
在这个例子中,当`MyString str1 = "Hello";` 或 `str1 = str2;` 被执行时,实际上是调用了上述定义的`operator=`。
相关问题
编写字符串运算符“+=”的重载函数,使运算符“+=”用于字符串的拼接运算,实现字符串的S1+=S2操作运算。分别用友元函数和成员函数实现。
友元函数实现:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class String {
private:
char *str;
public:
String(const char *s = NULL) {
if (s == NULL) {
str = new char[1];
str[0] = '\0';
} else {
str = new char[strlen(s) + 1];
strcpy(str, s);
}
}
~String() {
delete[] str;
}
void display() const {
cout << str << endl;
}
friend String operator+=(String &s1, const String &s2); // 友元函数
};
String operator+=(String &s1, const String &s2) {
char *temp = new char[strlen(s1.str) + strlen(s2.str) + 1];
strcpy(temp, s1.str);
strcat(temp, s2.str);
delete[] s1.str;
s1.str = temp;
return s1;
}
int main() {
String s1("Hello, ");
String s2("world!");
s1 += s2;
s1.display();
return 0;
}
```
成员函数实现:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class String {
private:
char *str;
public:
String(const char *s = NULL) {
if (s == NULL) {
str = new char[1];
str[0] = '\0';
} else {
str = new char[strlen(s) + 1];
strcpy(str, s);
}
}
~String() {
delete[] str;
}
void display() const {
cout << str << endl;
}
String &operator+=(const String &s) { // 成员函数
char *temp = new char[strlen(str) + strlen(s.str) + 1];
strcpy(temp, str);
strcat(temp, s.str);
delete[] str;
str = temp;
return *this;
}
};
int main() {
String s1("Hello, ");
String s2("world!");
s1 += s2;
s1.display();
return 0;
}
```
@全体成员 今天作业:编写字符串运算符“+=”的重载函数,使运算符“+=”用于字符串的拼接运算,实现字符串的S1+=S2操作运算。分别用友元函数和成员函数实现。
好的,这是一道关于C++中字符串运算符重载的编程作业。要求实现字符串的拼接运算,即将一个字符串S2添加到另一个字符串S1的末尾,实现S1+=S2的操作运算。
以下是友元函数实现的代码示例:
```
#include <iostream>
#include <cstring>
using namespace std;
class String {
char* str;
int size;
public:
String() {
size = 0;
str = new char[size+1];
str[0] = '\0';
}
String(char* s) {
size = strlen(s);
str = new char[size+1];
strcpy(str, s);
}
friend String operator+=(String &s1, String &s2);
void display() {
cout << str << endl;
}
};
String operator+=(String &s1, String &s2) {
char* temp = new char[s1.size + s2.size + 1];
strcpy(temp, s1.str);
strcat(temp, s2.str);
s1.size += s2.size;
delete [] s1.str;
s1.str = temp;
return s1;
}
int main() {
String s1("Hello");
String s2(" World!");
s1 += s2;
s1.display();
return 0;
}
```
以下是成员函数实现的代码示例:
```
#include <iostream>
#include <cstring>
using namespace std;
class String {
char* str;
int size;
public:
String() {
size = 0;
str = new char[size+1];
str[0] = '\0';
}
String(char* s) {
size = strlen(s);
str = new char[size+1];
strcpy(str, s);
}
String operator+=(String &s) {
char* temp = new char[size + s.size + 1];
strcpy(temp, str);
strcat(temp, s.str);
size += s.size;
delete [] str;
str = temp;
return *this;
}
void display() {
cout << str << endl;
}
};
int main() {
String s1("Hello");
String s2(" World!");
s1 += s2;
s1.display();
return 0;
}
```
以上两个代码示例都可以实现字符串的拼接运算,使用了不同的实现方式。友元函数的方式可以直接访问类的私有成员变量,而成员函数实现则需要使用this指针来访问类的成员变量。
阅读全文
相关推荐
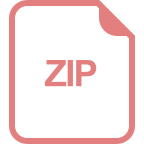
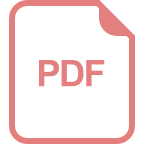
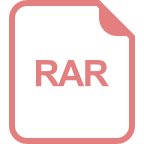
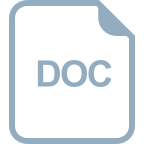







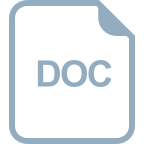
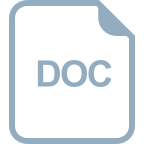
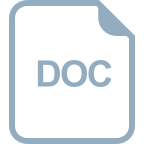
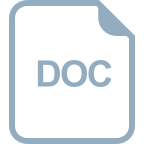
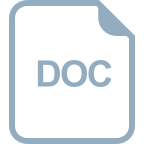
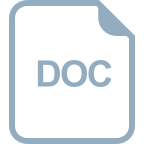
