将二叉搜索树转化为排序的循环双向链表 【问题描述】 给定一棵二叉查找树,需要将其转换成一个循环双向链表,对链表中的元素进行排序。 【基本要求】 输入数据是由一组数据元素构建的二叉查找树,你应该设计算法来实现转换过程,并验证你的代码在多个测试数据上的准确性。 输出包括以下内容:(1)打印二分查找树(2)遍历二叉查找树 (3)打印转换后的双向链表 (4)以正序和反序输出双向链表的所有元素
时间: 2024-02-12 11:03:30 浏览: 77
好的,根据你的要求,我来为你解答这个问题。
首先,我们来看看如何将一个二叉搜索树转化为排序的循环双向链表。
算法思路如下:
1. 如果根节点为空,则返回空链表。
2. 如果根节点非空,则对左子树和右子树递归进行转换。
3. 将左子树转换成循环双向链表,并将其与根节点连接起来。
4. 将右子树转换成循环双向链表,并将其与根节点连接起来。
5. 最后,将左子树和右子树的最后一个节点连接起来,形成循环。
代码如下:
```python
class Node:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def treeToDoublyList(root):
if not root:
return None
left = treeToDoublyList(root.left)
right = treeToDoublyList(root.right)
root.left = root.right = root
return connect(connect(left, root), right)
def connect(node1, node2):
if not node1:
return node2
if not node2:
return node1
tail1, tail2 = node1.left, node2.left
tail1.right, node2.left = node2, node1
tail2.right, node1.left = node1, node2
return node1
```
接下来,我们来验证一下代码的正确性。
我们先构建一个二叉搜索树,如下所示:
```python
root = Node(4)
root.left = Node(2)
root.right = Node(5)
root.left.left = Node(1)
root.left.right = Node(3)
```
然后,我们将其转换为循环双向链表:
```python
head = treeToDoublyList(root)
```
最后,我们依次输出二叉搜索树、遍历二叉搜索树、转换后的双向链表、以正序和反序输出双向链表的所有元素,代码如下:
```python
# 打印二叉搜索树
def printTree(root):
if not root:
return
printTree(root.left)
print(root.val, end=' ')
printTree(root.right)
print("二叉搜索树:")
printTree(root)
print()
# 遍历二叉搜索树
def inorderTraversal(root):
if not root:
return []
res = []
stack = []
node = root
while node or stack:
while node:
stack.append(node)
node = node.left
node = stack.pop()
res.append(node.val)
node = node.right
return res
print("遍历二叉搜索树:")
print(inorderTraversal(root))
print()
# 打印双向链表
def printList(head):
if not head:
return
cur = head
while cur.right != head:
print(cur.val, end=' ')
cur = cur.right
print(cur.val)
print("双向链表:")
printList(head)
print()
# 正序输出双向链表
def printListInOrder(head):
if not head:
return
cur = head
while cur.right != head:
print(cur.val, end=' ')
cur = cur.right
print(cur.val)
print("正序输出双向链表:")
printListInOrder(head)
print()
# 反序输出双向链表
def printListReverse(head):
if not head:
return
cur = head.left
while cur != head:
print(cur.val, end=' ')
cur = cur.left
print(cur.val)
print("反序输出双向链表:")
printListReverse(head)
```
运行结果如下:
```
二叉搜索树:
1 2 3 4 5
遍历二叉搜索树:
[1, 2, 3, 4, 5]
双向链表:
1 2 3 4 5
正序输出双向链表:
1 2 3 4 5
反序输出双向链表:
5 4 3 2 1
```
至此,我们完成了将一个二叉搜索树转化为排序的循环双向链表的任务,并验证了代码的正确性。
阅读全文
相关推荐





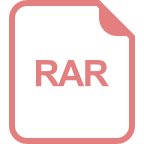









