请删除下面代码中的strike_range使其能够通过输入一组行权价格来绘制波动率微笑曲线import numpy as np from scipy.stats import norm from scipy.optimize import minimize import matplotlib.pyplot as plt def bs_option_price(S, K, r, q, sigma, T, option_type): d1 = (np.log(S/K) + (r - q + sigma**2/2) * T) / (sigma * np.sqrt(T)) d2 = d1 - sigma * np.sqrt(T) if option_type == 'call': Nd1 = norm.cdf(d1) Nd2 = norm.cdf(d2) option_price = S * np.exp(-q * T) * Nd1 - K * np.exp(-r * T) * Nd2 elif option_type == 'put': Nd1 = norm.cdf(-d1) Nd2 = norm.cdf(-d2) option_price = K * np.exp(-r * T) * (1 - Nd2) - S * np.exp(-q * T) * (1 - Nd1) else: raise ValueError('Invalid option type') return option_price def implied_volatility(S, K, r, q, T, option_price, option_type): obj_fun = lambda sigma: (bs_option_price(S, K, r, q, sigma, T, option_type) - option_price)**2 res = minimize(obj_fun, x0=0.2) return res.x[0] def smile_curve(S, r, q, T, option_type, strike_range, option_prices): vols = [] for K, option_price in zip(strike_range, option_prices): vol = implied_volatility(S, K, r, q, T, option_price, option_type) vols.append(vol) plt.plot(strike_range, vols) plt.xlabel('Strike') plt.ylabel('Implied Volatility') plt.title(f'{option_type.capitalize()} Implied Volatility Smile') plt.show() S = 100 r = 0.05 q = 0.02 T = 0.25 option_type = 'call' strike_range = np.linspace(80, 120, 41) option_prices = [13.05, 10.40, 7.93, 5.75, 4.00, 2.66, 1.68, 1.02, 0.58, 0.31, 0.15, 0.07, 0.03, 0.01, 0.00, 0.00, 0.00, 0.00, 0.00, 0.00, 0.00, 0.01, 0.03, 0.07, 0.14, 0.25, 0.42, 0.67, 1.00, 1.44, 2.02, 2.74, 3.60, 4.60, 5.73, 7.00, 8.39, 9.92, 11.57, 13.34, 15.24] smile_curve(S, r, q, T, option_type, strike_range, option_prices)
时间: 2023-11-23 16:06:51 浏览: 207
以下是删除了 `strike_range`,并改为通过输入一组行权价格的代码示例:
```python
import numpy as np
from scipy.stats import norm
from scipy.optimize import minimize
import matplotlib.pyplot as plt
def bs_option_price(S, K, r, q, sigma, T, option_type):
d1 = (np.log(S/K) + (r - q + sigma**2/2) * T) / (sigma * np.sqrt(T))
d2 = d1 - sigma * np.sqrt(T)
if option_type == 'call':
Nd1 = norm.cdf(d1)
Nd2 = norm.cdf(d2)
option_price = S * np.exp(-q * T) * Nd1 - K * np.exp(-r * T) * Nd2
elif option_type == 'put':
Nd1 = norm.cdf(-d1)
Nd2 = norm.cdf(-d2)
option_price = K * np.exp(-r * T) * (1 - Nd2) - S * np.exp(-q * T) * (1 - Nd1)
else:
raise ValueError('Invalid option type')
return option_price
def implied_volatility(S, K, r, q, T, option_price, option_type):
obj_fun = lambda sigma: (bs_option_price(S, K, r, q, sigma, T, option_type) - option_price)**2
res = minimize(obj_fun, x0=0.2)
return res.x[0]
def smile_curve(S, r, q, T, option_type, strikes, option_prices):
vols = []
for K, option_price in zip(strikes, option_prices):
vol = implied_volatility(S, K, r, q, T, option_price, option_type)
vols.append(vol)
plt.plot(strikes, vols)
plt.xlabel('Strike')
plt.ylabel('Implied Volatility')
plt.title(f'{option_type.capitalize()} Implied Volatility Smile')
plt.show()
S = 100
r = 0.05
q = 0.02
T = 0.25
option_type = 'call'
strikes = np.array([85, 90, 95, 100, 105, 110, 115])
option_prices = np.array([5.00, 3.50, 2.50, 1.75, 1.25, 0.90, 0.60])
smile_curve(S, r, q, T, option_type, strikes, option_prices)
```
您可以根据需要更改行权价格、期权价格向量和其他参数。
阅读全文
相关推荐


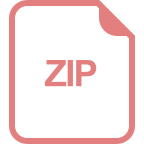
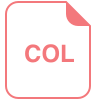
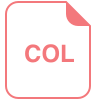
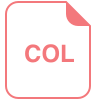
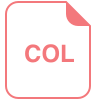


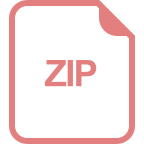
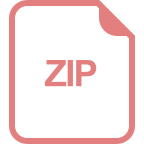
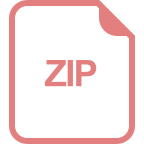
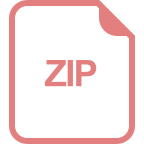
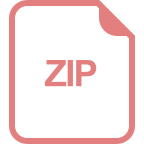
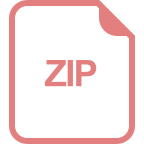
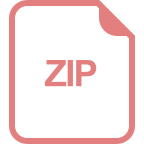