shape【1】在Python中什么意思,给出具体的代码示例
时间: 2023-06-13 22:08:56 浏览: 57
在Python中,`shape[1]`通常是用来获取数组或矩阵的列数(第二个维度的大小)。
具体的代码示例如下:
```python
import numpy as np
# 创建一个2x3的矩阵
matrix = np.array([[1, 2, 3], [4, 5, 6]])
# 获取矩阵的列数
num_cols = matrix.shape[1]
# 输出列数
print(num_cols) # 输出 3
```
在上面的示例中,`shape[1]`用于获取矩阵的列数,即第二个维度的大小,这里是3。
相关问题
什么是多步K-均值聚类并给出python代码示例
多步K-均值聚类是一种基于迭代的聚类算法,其核心思想是通过多次应用K-均值聚类来优化聚类结果。下面是一个使用Python实现多步K-均值聚类的示例代码:
```python
import numpy as np
from sklearn.cluster import KMeans
def multi_kmeans(X, n_clusters, n_steps):
"""
多步K-均值聚类
:param X: 输入数据,shape为[n_samples, n_features]
:param n_clusters: 聚类数量
:param n_steps: 迭代次数
:return: 聚类结果,shape为[n_samples,]
"""
labels = np.zeros(X.shape[0])
for i in range(n_steps):
kmeans = KMeans(n_clusters=n_clusters, random_state=i)
kmeans.fit(X)
new_labels = kmeans.predict(kmeans.cluster_centers_)
for j in range(n_clusters):
labels[kmeans.labels_ == j] = new_labels[j]
return labels.astype(int)
```
上述代码中,我们使用了scikit-learn库中的KMeans类实现了单次K-均值聚类,并在此基础上进行了多步迭代。具体来说,我们在每次迭代中,使用KMeans类聚类得到聚类中心,并将每个聚类中心作为新的数据点再次进行聚类。最终,我们将每个样本点归入到最终的聚类中心中。
python OpenCV根据畸变半径进行桶形矫正给出示例代码
下面是一个简单的Python示例代码,根据畸变半径进行桶形矫正:
```python
import cv2
import numpy as np
# 读取图像
img = cv2.imread('example.jpg')
# 计算畸变半径
h, w = img.shape[:2]
distCoeff = np.zeros((4,1))
k1 = -0.5
k2 = 0.25
p1 = 0.0
p2 = 0.0
distCoeff[0,0] = k1
distCoeff[1,0] = k2
distCoeff[2,0] = p1
distCoeff[3,0] = p2
focal_length = w
center = (w/2, h/2)
camera_matrix = np.array([[focal_length, 0, center[0]],
[0, focal_length, center[1]],
[0, 0, 1]], dtype = "double")
new_camera_matrix, roi = cv2.getOptimalNewCameraMatrix(camera_matrix, distCoeff, (w,h), 1, (w,h))
# 进行桶形矫正
mapx, mapy = cv2.initUndistortRectifyMap(camera_matrix, distCoeff, None, new_camera_matrix, (w,h), 5)
dst = cv2.remap(img, mapx, mapy, cv2.INTER_LINEAR)
# 显示原图和矫正后的图像
cv2.imshow('Original', img)
cv2.imshow('Corrected', dst)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个示例代码中,我们首先读取了一张图像,然后使用OpenCV中的`cv2.getOptimalNewCameraMatrix`函数计算出新的相机矩阵和ROI区域。接下来,我们使用`cv2.initUndistortRectifyMap`函数计算出畸变矫正的映射表,然后使用`cv2.remap`函数将原始图像映射到畸变矫正后的图像。最后,我们显示原始图像和矫正后的图像。
相关推荐
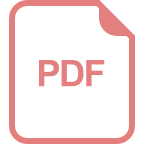
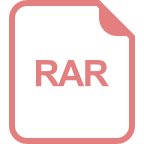
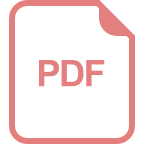












