C语言不用<math.h>里所包含的函数
时间: 2023-08-11 09:04:09 浏览: 94
好的,如果你不想使用 `math.h` 中的库函数,那么可以尝试使用以下代码:
```c
#include <stdio.h>
int main()
{
float money, year, rate, interest;
printf("Enter money, year and rate: ");
scanf("%f %f %f", &money, &year, &rate);
interest = money * (1 + rate) * year - money;
printf("interest = %.2f", interest);
return 0;
}
```
这个代码使用 `scanf` 函数读入输入数据,然后根据公式计算利息,最后使用 `printf` 函数输出结果。注意,为了保留两位小数,我们在输出时使用了 `.2f` 的格式控制符。
相关问题
c语言中#include <math.h>
在C语言中,`#include <math.h>`是一个预处理指令,用于引入C标准库中的数学函数。这个头文件包含了数学运算相关的函数,使得程序员能够方便地在程序中使用诸如三角函数(sin, cos, tan)、指数和对数函数(exp, log, pow)、浮点数运算(sqrt, floor, ceil)以及其他数学操作。
当你在代码中包含`<math.h>`后,你可以直接使用其中定义的函数,例如计算圆周率π可以用`M_PI`常量,执行平方根计算可以用`sqrt()`函数,等等。例如:
```c
#include <math.h>
double radius = 5.0;
double area = M_PI * pow(radius, 2);
```
C语言中<math.h>的函数pow()函数的用法
在C语言中,《math.h》头文件包含了许多数学运算相关的函数,其中`pow()`函数用于计算一个数的幂次。它的基本用法如下:
```c
double pow(double base, double exponent);
```
`pow(base, exponent)`接受两个`double`类型的参数:
1. `base`:底数,即乘方运算中的第一个数。
2. `exponent`:指数,即乘方运算中的第二个数。
这个函数会返回`base`的`exponent`次方的结果。例如,`pow(2.0, 3.0)`将返回8.0。
如果你需要处理整数幂,可以先将指数转换成`double`,因为`pow()`默认处理的是实数幂。如果只对整数幂感兴趣,并且想保持效率,可以使用`**`操作符或`exp()`函数配合`log10()`函数。
示例代码:
```c
#include <stdio.h>
#include <math.h>
int main() {
double result = pow(2.0, 3.0);
printf("2 to the power of 3 is: %.2lf\n", result);
// 或者处理整数幂
int base = 5;
long int exponent = 4;
result = pow((double)base, exponent);
printf("%d raised to %ld is: %.2lf\n", base, exponent, result);
return 0;
}
```
阅读全文
相关推荐
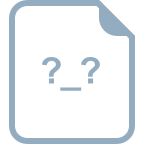
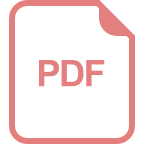
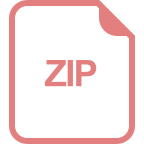













