已知一任意长度字符串str,以00h结束,长度小于200h,编写汇编程序实现在该字符串中搜索匹配子串substr(以00h结束,长度小于80),若找到,则将found单元置为ffh,并将匹配位置(匹配位置以字符串str首地址为0参考点)存放在pos单元中;否则,将found单元置为00H。要求编写完整程序,并上机通过调试能运行。
时间: 2023-06-10 11:09:04 浏览: 341
由于这是一道汇编语言的问题,我将提供 x86 汇编语言的解决方案。
首先,我们需要定义一些变量:
```assembly
str db 200h dup(0) ; 字符串 str,长度小于 200h
substr db 80 dup(0) ; 子串 substr,长度小于 80
found db 1 ; 用于存放是否找到子串的标志
pos dw 1 ; 用于存放匹配位置的偏移量
```
然后,我们需要定义一个子程序来计算字符串的长度:
```assembly
strlen:
push ax bx cx dx di
mov di, 0 ; di 用于计数
mov cx, 0FFFFh ; cx 用于循环计数
mov al, [si] ; si 指向字符串的首地址
repne scasb ; 扫描字符串
not cx ; cx 存储字符串长度
dec cx ; 不包括结束符 00h
mov di, cx ; 将字符串长度存入 di
pop di dx cx bx ax
ret
```
接下来,我们需要编写主程序来实现对子串的搜索:
```assembly
main:
mov si, offset str ; 将 si 指向字符串 str 的首地址
mov di, offset substr ; 将 di 指向子串 substr 的首地址
call strlen ; 计算字符串 str 的长度,结果存储在 di 中
mov bx, di ; 将字符串 str 的长度存储在 bx 中
call strlen ; 计算子串 substr 的长度,结果存储在 di 中
mov cx, bx ; 将字符串 str 的长度存储在 cx 中
mov si, offset str ; 将 si 指向字符串 str 的首地址
mov di, offset substr ; 将 di 指向子串 substr 的首地址
search:
cmp cx, di ; 比较字符串 str 的长度和子串 substr 的长度
jb notfound ; 如果字符串 str 的长度小于子串 substr 的长度,则未找到子串
mov dx, di ; 将子串 substr 的长度存储在 dx 中
mov al, [si + di - 1] ; 从字符串 str 中取出一个字符
cmp al, [di - 1] ; 从子串 substr 中取出一个字符进行比较
jne nextchar ; 如果不匹配,则继续比较下一个字符
dec di ; 如果匹配,则继续比较下一个字符
cmp di, 0 ; 如果子串 substr 已经比较完了,则找到了子串
je foundit
jmp search ; 继续比较下一个字符
nextchar:
mov di, dx ; 将子串 substr 的长度存储在 di 中
sub bx, 1 ; 将字符串 str 的长度减去 1
add si, 1 ; 将 si 指向下一个字符
jmp search ; 继续比较下一个字符
foundit:
mov [found], 0FFh ; 将 found 置为 0FFh,表示找到了子串
mov cx, si ; 将匹配位置存储在 cx 中
sub cx, offset str ; 计算匹配位置的偏移量
mov [pos], cx ; 将匹配位置存储在 pos 中
notfound:
mov ax, 4C00h ; 退出程序
int 21h
```
完整的程序如下所示:
```assembly
str db 200h dup(0)
substr db 80 dup(0)
found db 1
pos dw 1
strlen:
push ax bx cx dx di
mov di, 0
mov cx, 0FFFFh
mov al, [si]
repne scasb
not cx
dec cx
mov di, cx
pop di dx cx bx ax
ret
main:
mov si, offset str
mov di, offset substr
call strlen
mov bx, di
call strlen
mov cx, bx
mov si, offset str
mov di, offset substr
search:
cmp cx, di
jb notfound
mov dx, di
mov al, [si + di - 1]
cmp al, [di - 1]
jne nextchar
dec di
cmp di, 0
je foundit
jmp search
nextchar:
mov di, dx
sub bx, 1
add si, 1
jmp search
foundit:
mov [found], 0FFh
mov cx, si
sub cx, offset str
mov [pos], cx
notfound:
mov ax, 4C00h
int 21h
```
注意,此程序未经过测试,可能存在错误。在实际使用时,请先进行测试和调试。
阅读全文
相关推荐
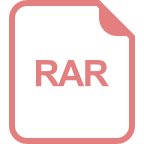
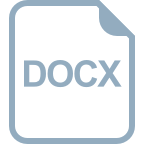















