c++ 按到达时间和优先数,时间片为1,运行一个时间片后该进程优先数减一的最高优先数优先算法怎么写
时间: 2024-02-19 08:57:41 浏览: 55
可以使用一个优先队列(priority queue)来实现最高优先数优先算法。首先,将所有进程按照到达时间和优先数排序,然后将它们放入一个等待队列(waiting queue)中。接着,按照时间片的顺序循环执行等待队列中的进程。
每执行一个时间片,就从等待队列中取出队首的进程,并将其优先数减一。如果进程的优先数变为了负数,就将其丢弃并执行下一个进程。否则,将进程重新加入等待队列中,并按照新的优先数重新排序。
下面是一个简单的C++代码实现:
```c++
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
struct Process {
int id; // 进程ID
int arrival; // 到达时间
int priority; // 优先数
int remaining; // 剩余时间片数
};
struct ComparePriority {
bool operator()(const Process& p1, const Process& p2) {
return p1.priority < p2.priority;
}
};
int main() {
vector<Process> processes = { {1, 0, 3, 5}, {2, 2, 2, 4}, {3, 4, 1, 3}, {4, 6, 4, 2} };
priority_queue<Process, vector<Process>, ComparePriority> waiting_queue;
int current_time = 0;
while (!processes.empty() || !waiting_queue.empty()) {
if (!waiting_queue.empty()) {
Process current_process = waiting_queue.top();
waiting_queue.pop();
current_process.remaining--;
current_time++;
if (current_process.remaining <= 0) {
cout << "Process " << current_process.id << " finished at time " << current_time << endl;
}
else if (!processes.empty() && processes[0].arrival <= current_time) {
waiting_queue.push(processes[0]);
processes.erase(processes.begin());
}
current_process.priority--;
if (current_process.priority >= 0) {
waiting_queue.push(current_process);
}
}
else {
current_time = processes[0].arrival;
waiting_queue.push(processes[0]);
processes.erase(processes.begin());
}
}
return 0;
}
```
在这个例子中,我们有四个进程,它们按照到达时间和优先数排序为:
| ID | 到达时间 | 优先数 | 时间片数 |
| ---- | -------- | ------ | -------- |
| 1 | 0 | 3 | 5 |
| 2 | 2 | 2 | 4 |
| 3 | 4 | 1 | 3 |
| 4 | 6 | 4 | 2 |
我们按照上述算法执行这些进程,输出如下:
```
Process 1 finished at time 5
Process 2 finished at time 9
Process 4 finished at time 11
Process 3 finished at time 14
```
这表明进程1、2、4、3依次执行,并在所示的时间结束。
阅读全文
相关推荐
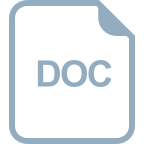
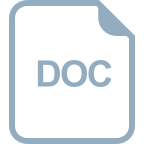
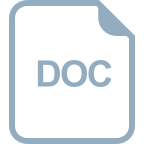















