osg鼠标点击获取经纬度
时间: 2023-08-28 14:06:41 浏览: 83
要获取鼠标点击位置的经纬度,你可以使用以下步骤:
1. 设置一个 osgGA::GUIEventHandler 的事件处理器,通过重载其 handle 方法来处理鼠标点击事件。例如:
```cpp
class MouseClickHandler : public osgGA::GUIEventHandler
{
public:
virtual bool handle(const osgGA::GUIEventAdapter& ea, osgGA::GUIActionAdapter& aa)
{
if (ea.getEventType() == osgGA::GUIEventAdapter::RELEASE && ea.getButton() == osgGA::GUIEventAdapter::LEFT_MOUSE_BUTTON)
{
osgViewer::View* view = dynamic_cast<osgViewer::View*>(&aa);
if (view)
{
osg::ref_ptr<osgUtil::LineSegmentIntersector> intersector = new osgUtil::LineSegmentIntersector(osgUtil::Intersector::WINDOW, ea.getX(), ea.getY());
osgUtil::IntersectionVisitor iv(intersector.get());
view->getCamera()->accept(iv);
if (intersector->containsIntersections())
{
const osgUtil::LineSegmentIntersector::Intersection& intersection = *(intersector->getIntersections().begin());
osg::Vec3d point = intersection.getWorldIntersectPoint();
osg::Vec3d latLonAlt;
osg::ref_ptr<osgEarth::MapNode> mapNode = osgEarth::MapNode::findMapNode(view->getSceneData());
if (mapNode.valid() && mapNode->getMap()->getProfile()->getSRS()->transformFromWorld(point, latLonAlt))
{
double latitude = osg::DegreesToRadians(latLonAlt.y());
double longitude = osg::DegreesToRadians(latLonAlt.x());
// Do something with latitude and longitude...
}
}
}
}
return false;
}
};
```
2. 在 handle 方法中,使用 osgUtil::LineSegmentIntersector 类来获取鼠标点击位置的世界坐标点。例如:
```cpp
osg::ref_ptr<osgUtil::LineSegmentIntersector> intersector = new osgUtil::LineSegmentIntersector(osgUtil::Intersector::WINDOW, ea.getX(), ea.getY());
osgUtil::IntersectionVisitor iv(intersector.get());
view->getCamera()->accept(iv);
if (intersector->containsIntersections())
{
const osgUtil::LineSegmentIntersector::Intersection& intersection = *(intersector->getIntersections().begin());
osg::Vec3d point = intersection.getWorldIntersectPoint();
// Do something with the point...
}
```
3. 如果你的场景使用了 osgEarth 地球引擎,那么你可以使用 osgEarth::MapNode 类来将世界坐标点转换为经纬度坐标。例如:
```cpp
osg::Vec3d latLonAlt;
osg::ref_ptr<osgEarth::MapNode> mapNode = osgEarth::MapNode::findMapNode(view->getSceneData());
if (mapNode.valid() && mapNode->getMap()->getProfile()->getSRS()->transformFromWorld(point, latLonAlt))
{
double latitude = osg::DegreesToRadians(latLonAlt.y());
double longitude = osg::DegreesToRadians(latLonAlt.x());
// Do something with latitude and longitude...
}
```
以上就是获取鼠标点击位置的经纬度的基本步骤。需要注意的是,这里的经纬度使用的是弧度制,如果需要使用角度制,可以使用 osg::RadiansToDegrees 函数进行转换。
相关推荐
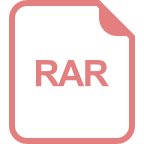










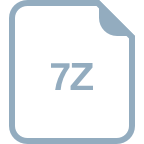