python 检测卷纸带缠绕圆柱是否整齐的程序
时间: 2024-05-10 11:20:31 浏览: 93
以下是一个简单的Python程序,可以检测卷纸带缠绕圆柱是否整齐:
```python
import math
def is_cylinder_evenly_wound(diameter, width, thickness):
circumference = math.pi * diameter
layers = math.ceil(thickness / width)
layer_thickness = thickness / layers
for i in range(layers):
layer_radius = (diameter / 2) - (i * layer_thickness)
layer_circumference = 2 * math.pi * layer_radius
if abs(layer_circumference - circumference) > 0.01:
return False
return True
```
该函数接受三个参数:圆柱直径、卷带宽度和卷带厚度。它首先计算每层的厚度,然后根据层数计算每个层的半径。最后,它检查每个层的周长是否与圆柱周长相等(在0.01的误差范围内)。
如果圆柱是整齐缠绕的,则该函数将返回True,否则返回False。
相关问题
opencv 检测卷纸带缠绕圆柱是否整齐的程序
以下是使用OpenCV检测卷纸带缠绕圆柱是否整齐的程序:
1. 导入OpenCV库和numpy库
```python
import cv2
import numpy as np
```
2. 读取图片并转换为灰度图像
```python
img = cv2.imread('image.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
3. 进行Canny边缘检测
```python
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
```
4. 进行霍夫圆检测
```python
circles = cv2.HoughCircles(edges, cv2.HOUGH_GRADIENT, 1, 20, param1=50, param2=30, minRadius=0, maxRadius=0)
```
其中,参数param1和param2控制霍夫圆检测算法的敏感度和精度,minRadius和maxRadius控制检测到的圆的半径范围。
5. 判断圆是否整齐
如果检测到多个圆,则需要判断它们是否整齐。判断方法可以是计算圆心之间的距离,如果距离差异小于某个阈值,则认为它们整齐。
```python
if circles is not None:
circles = np.round(circles[0, :]).astype("int")
if len(circles) > 1:
distances = []
for i in range(len(circles)):
for j in range(i + 1, len(circles)):
distance = np.sqrt((circles[i][0] - circles[j][0]) ** 2 + (circles[i][1] - circles[j][1]) ** 2)
distances.append(distance)
if np.std(distances) < 10:
print("The circles are neat.")
else:
print("The circles are not neat.")
else:
print("No circles detected.")
```
以上代码中,如果检测到多个圆,则计算圆心之间的距离,并计算它们的标准差,如果标准差小于10,则认为它们整齐,否则认为它们不整齐。如果只检测到一个圆,则输出“未检测到圆”。
阅读全文
相关推荐



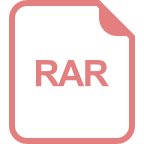


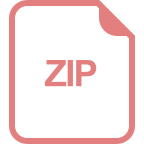
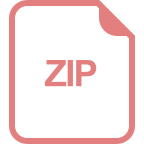





