用C写一个串口接收,使用环形队列缓冲
时间: 2023-09-06 07:05:00 浏览: 63
### 回答1:
我可以给你一个简单的示例程序: #include <stdio.h>
#include <string.h>
#include <stdlib.h>#define BUFFER_SIZE 8typedef struct {
unsigned char buffer[BUFFER_SIZE];
int head;
int tail;
int count;
} RingBuffer;// 初始化环形队列
void init_ring_buffer(RingBuffer *rb)
{
memset(rb->buffer, 0, BUFFER_SIZE);
rb->head = 0;
rb->tail = 0;
rb->count = 0;
}// 向环形队列放入数据
int put_data(RingBuffer *rb, unsigned char data)
{
if (rb->count == BUFFER_SIZE) {
return -1;
}
rb->buffer[rb->tail] = data;
rb->tail = (rb->tail + 1) % BUFFER_SIZE;
rb->count++;
return 0;
}// 从环形队列取出数据
int get_data(RingBuffer *rb, unsigned char *data)
{
if (rb->count == 0) {
return -1;
}
*data = rb->buffer[rb->head];
rb->head = (rb->head + 1) % BUFFER_SIZE;
rb->count--;
return 0;
}int main(void)
{
// 使用环形队列缓冲串口接收的数据
RingBuffer rb;
init_ring_buffer(&rb); // 串口接收到的数据
unsigned char data; // 将接收到的数据放入环形队列
put_data(&rb, data); // 从环形队列中取出数据
get_data(&rb, &data); return 0;
}
### 回答2:
串口是计算机与外设之间传送数据的接口,而串口接收则是指计算机从外设读取数据的过程。使用C语言编写一个串口接收程序,可以采用环形队列缓冲来实现。环形队列是一种数据结构,可以循环利用内存空间,在串口接收中,可以用来存储接收到的数据。
首先,在C语言中定义一个环形队列结构体,包含有缓冲区、读指针和写指针等成员。缓冲区用来存储接收到的数据,读指针指向下一个要读取的数据,写指针指向下一个要写入的数据。
在串口接收程序中,可以使用串口中断来触发数据接收。当接收到数据时,将数据写入环形队列的缓冲区中,同时写指针向后移动一位。如果写指针达到缓冲区的末尾,可以将其设置为缓冲区的起始位置,实现环形队列的循环利用。
如果要读取数据,可以通过读取读指针所指向的数据,并将读指针向后移动一位。如果读指针达到缓冲区的末尾,同样也可以将其设置为缓冲区的起始位置。
通过使用环形队列缓冲,可以实现串口接收程序的高效运行。因为环形队列可以循环利用内存空间,避免了数据的复制和移动,提高了数据的读写效率。另外,环形队列还可以解决数据溢出的问题,当缓冲区已满时,可以选择丢弃最旧的数据,保证程序的稳定性和可靠性。
总结起来,使用C语言编写一个串口接收程序,可以通过环形队列缓冲来实现,提高数据读写效率和程序的可靠性。
### 回答3:
使用C语言编写串口接收程序,可以通过环形队列缓冲来处理接收到的数据。具体步骤如下:
1. 定义环形队列的数据结构:
```c
#define QUEUE_SIZE 100 // 环形队列的大小
typedef struct {
char buffer[QUEUE_SIZE];
int front; // 队列头索引
int rear; // 队列尾索引
int count; // 队列中元素数量
} CircularQueue;
```
2. 初始化环形队列:
```c
void initQueue(CircularQueue *queue) {
queue->front = 0;
queue->rear = 0;
queue->count = 0;
}
```
3. 定义串口数据接收函数:
```c
void receiveData(CircularQueue *queue) {
char data;
// 假设有一个函数 SerialGetChar() 用于从串口读取一个字符
while (SerialGetChar(&data)) {
if (queue->count < QUEUE_SIZE) {
queue->buffer[queue->rear] = data;
queue->rear = (queue->rear + 1) % QUEUE_SIZE;
queue->count++;
} else {
// 队列已满,无法继续接收数据
break;
}
}
}
```
4. 使用接收到的数据:
```c
void processData(CircularQueue *queue) {
while (queue->count > 0) {
char data = queue->buffer[queue->front];
// 处理接收到的数据,例如打印到终端
printf("%c", data);
queue->front = (queue->front + 1) % QUEUE_SIZE;
queue->count--;
}
}
```
5. 主程序中调用以上函数:
```c
int main() {
CircularQueue queue;
initQueue(&queue);
while (1) {
receiveData(&queue);
processData(&queue);
}
return 0;
}
```
通过以上步骤,可以实现一个使用环形队列缓冲的串口接收程序。程序通过循环不断从串口读取数据,将数据存储到环形队列中,并在需要使用数据时从队列中取出进行处理。这样可以提高程序接收数据的效率,避免数据丢失和冲突。
相关推荐
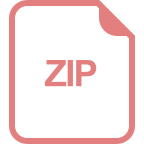
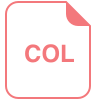
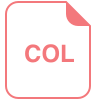
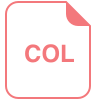
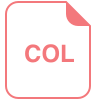
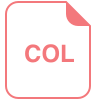








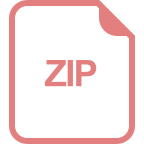