补全代码Status EnQueue(SqQueue *Q, QElemType e) { }
时间: 2024-05-29 09:10:30 浏览: 18
Status EnQueue(SqQueue *Q, QElemType e) {
if (Q->rear == MAXSIZE) { // 队列已满
return ERROR;
}
Q->data[Q->rear] = e; // 将新元素添加到队列尾部
Q->rear++; // 队列尾指针后移
return OK;
}
相关问题
补全代码Status EnQueue(LinkQueue *Q, QElemType e) { }
Status EnQueue(LinkQueue *Q, QElemType e) {
QueuePtr p = (QueuePtr)malloc(sizeof(QNode)); // 创建一个新节点
if (!p) {
exit(OVERFLOW); // 内存分配失败
}
p->data = e; // 将元素e赋值给新节点的data域
p->next = NULL; // 新节点的next指针置为NULL
Q->rear->next = p; // 将新节点插入到队尾
Q->rear = p; // 修改队尾指针
return OK;
}
#include<stdio.h> typedef int QElemType;//定义队列元素为int类型,提前换个名称适合后期对程序修改 typedef int Status; #define MAXSIZE 11 #define OK 1 #define ERROR 0 typedef struct { QElemType data[MAXSIZE]; int front;//定义头指针 int rear;//定义尾指针,若队列不空,指向队列尾元素的下一个位置 }sqQueue; //初始化一个空队列Q Status InitQueue(sqQueue *Q) { Q->front=0; Q->rear=0; return OK; } //循环队列求队列长度 Status QueueLength(sqQueue Q) { return (Q.rear-Q.front+MAXSIZE)%MAXSIZE; } //若队列未满,则插入元素e为Q新的队尾元素 Status EnQueue(sqQueue *Q,QElemType e) { if((Q->rear+1)%MAXSIZE==Q->front)//判断队列是否满 ps:保留一个空余的位置也代表队列满 return ERROR; Q->data[Q->rear]=e;//将元素e赋给队尾------对Q->rear进行思考为啥不是Q->rear+1???与数组 Q->rear=(Q->rear+1)%MAXSIZE;//若在最后一个转到数值头部 return OK; } //若队列不空,则删除Q中队头元素,用e返回其值 Status DeQueue(sqQueue *Q,QElemType *e) { if(Q->front==Q->rear)//队列空的判断 { return ERROR; } *e=Q->data[Q->front]; Q->front=(Q->front+1)%MAXSIZE; } int main() { int m,n; sqQueue *Q; InitQueue(Q); printf("%d\n",QueueLength(*Q)); scanf("%d",&m); EnQueue(Q,m); printf("%d\n",QueueLength(*Q)); return 0; }
这段代码存在以下几个问题:
1. 在 main 函数中定义了一个指向 sqQueue 结构体的指针 Q,但没有为其分配内存空间,会导致程序崩溃。需要改为 `sqQueue Q;`。
2. 在 EnQueue 函数中,将元素 e 赋给队尾时,索引应该是 Q->rear,而不是 Q->rear+1。因为 Q->rear 指向的是队列尾元素的下一个位置,而不是队列尾元素本身。
3. 在 DeQueue 函数中,忘记了返回 OK,导致函数无法正常结束。需要在函数结尾添加 `return OK;`。
修改后的代码如下:
相关推荐
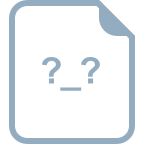
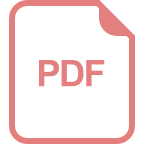
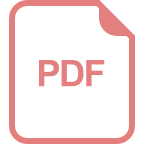












