hog = cv2.HOGDescriptor()效果不好
时间: 2024-04-03 09:36:29 浏览: 24
如果你觉得 `cv2.HOGDescriptor()` 的效果不好,可以尝试以下几种方法:
1. 调整参数:HOG 算法的效果与参数的设置有很大关系。你可以调整参数来尝试提高算法的效果,例如 `winSize`、`blockSize`、`cellSize`、`nbins` 等等。
2. 使用其他特征提取算法:除了 HOG 算法之外,还有很多其他的特征提取算法可以使用,例如 SIFT、SURF、ORB 等等。你可以尝试使用这些算法来提取图像特征。
3. 使用深度学习算法:深度学习算法在图像处理领域取得了很多成功的应用。你可以尝试使用一些深度学习算法来进行对象识别,例如卷积神经网络(CNN)等等。
4. 使用其他库:除了 OpenCV 之外,还有很多其他的计算机视觉库可以使用,例如 TensorFlow、PyTorch、Caffe 等等。你可以尝试使用这些库来进行对象识别。
相关问题
descriptor = cv2.HOGDescriptor() hog = descriptor.compute(gray)
这段代码使用了OpenCV库中的HOGDescriptor类来计算图像的HOG特征。HOG(Histogram of Oriented Gradients)特征是一种常用的图像特征,它可以描述图像中物体的形状和纹理信息。在这里,首先创建了一个HOGDescriptor对象,然后通过该对象的compute方法来计算输入图像gray的HOG特征,结果保存在hog变量中。
# 使用HOGDescriptor类计算HOG特征 descriptor = cv2.HOGDescriptor() hog = descriptor.compute(gray)
这段代码使用了OpenCV中的HOGDescriptor类来计算图像的HOG特征。具体来说,它首先创建了一个HOGDescriptor对象,然后使用该对象的compute()方法计算输入图像gray的HOG特征。最终结果保存在hog变量中。请注意,使用HOGDescriptor类需要先安装OpenCV库。
相关推荐
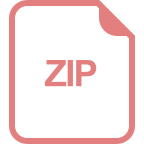
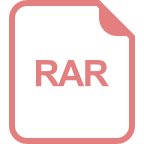
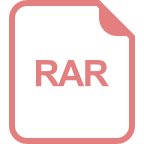












