c语言初始化一个数据元素为字符串类型的二叉树,并对其实现创建及求最大深度操作。二叉树的深度为根节点到最远叶子节点的最长路径上的节点数。
时间: 2024-05-10 19:17:59 浏览: 6
以下是C语言实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct TreeNode {
char data[20];
struct TreeNode *left;
struct TreeNode *right;
} TreeNode, *pTreeNode;
// 创建二叉树
void createTree(pTreeNode *root) {
char val[20];
printf("请输入节点的值(输入#表示空节点):");
scanf("%s", val);
if (strcmp(val, "#") == 0) { // 空节点
*root = NULL;
} else {
*root = (pTreeNode)malloc(sizeof(TreeNode));
strcpy((*root)->data, val); // 复制字符串
createTree(&(*root)->left);
createTree(&(*root)->right);
}
}
// 求二叉树的最大深度
int maxDepth(pTreeNode root) {
if (root == NULL) {
return 0;
} else {
int leftDepth = maxDepth(root->left);
int rightDepth = maxDepth(root->right);
return (leftDepth > rightDepth) ? (leftDepth + 1) : (rightDepth + 1);
}
}
int main() {
pTreeNode root;
createTree(&root);
int depth = maxDepth(root);
printf("最大深度为:%d\n", depth);
return 0;
}
```
以上代码实现了二叉树的创建和求最大深度操作。其中,`createTree()`函数实现了使用前序遍历方式输入各个节点的值,`maxDepth()`函数实现了递归地求解二叉树的最大深度。
相关推荐
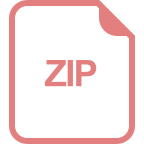
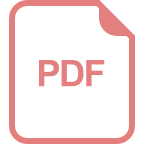














