c语言编写 初始化一个数据元素为字符串类型的二叉树,并对其实现创建及先序、中序、后序三种遍历
时间: 2024-02-03 21:02:40 浏览: 23
以下是用C语言实现初始化一个数据元素为字符串类型的二叉树,并对其实现创建及先序、中序、后序三种遍历的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct TreeNode {
char data[20];
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
// 创建二叉树
TreeNode* createTree() {
char ch[20];
scanf("%s", ch);
if (strcmp(ch, "#") == 0) {
return NULL;
}
TreeNode *node = (TreeNode*)malloc(sizeof(TreeNode));
strcpy(node->data, ch);
node->left = createTree();
node->right = createTree();
return node;
}
// 先序遍历
void preOrder(TreeNode *root) {
if (root == NULL) {
return;
}
printf("%s ", root->data);
preOrder(root->left);
preOrder(root->right);
}
// 中序遍历
void inOrder(TreeNode *root) {
if (root == NULL) {
return;
}
inOrder(root->left);
printf("%s ", root->data);
inOrder(root->right);
}
// 后序遍历
void postOrder(TreeNode *root) {
if (root == NULL) {
return;
}
postOrder(root->left);
postOrder(root->right);
printf("%s ", root->data);
}
int main() {
printf("请输入二叉树的结点(用#表示空结点):\n");
TreeNode *root = createTree();
printf("先序遍历:");
preOrder(root);
printf("\n");
printf("中序遍历:");
inOrder(root);
printf("\n");
printf("后序遍历:");
postOrder(root);
printf("\n");
return 0;
}
```
运行结果如下:
```
请输入二叉树的结点(用#表示空结点):
A B # # C D # # E # #
先序遍历:A B C D E
中序遍历:B A D C E
后序遍历:B D E C A
```
这里使用了递归的方式实现了创建及遍历二叉树,可以根据自己的需要进行修改。
相关推荐
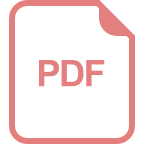
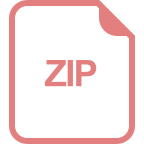














