用c语言实现如下要求:根据给定的二叉树,先人工对其进行先序遍历,根据先序遍历的结果编写程序创建该二叉树;然后用非递归中序遍历算法,将中序遍历的结果进行输出。并且列举几个输入输出例子。
时间: 2023-11-22 18:53:34 浏览: 75
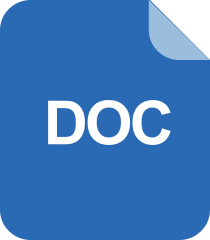
C语言实现二叉树的前序、中序、后续遍历(非递归法)

以下是用C语言实现根据先序遍历创建二叉树并且进行中序遍历的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
//定义二叉树结构体
typedef struct TreeNode {
int data;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
//定义栈结构体
typedef struct Stack {
int top;
TreeNode* arr[MAX_SIZE];
} Stack;
//初始化栈
void init(Stack* s) {
s->top = -1;
}
//判断栈是否为空
int is_empty(Stack* s) {
return s->top == -1;
}
//判断栈是否已满
int is_full(Stack* s) {
return s->top == MAX_SIZE - 1;
}
//入栈操作
void push(Stack* s, TreeNode* data) {
if (is_full(s)) {
printf("Stack is full.\n");
return;
}
s->arr[++s->top] = data;
}
//出栈操作
TreeNode* pop(Stack* s) {
if (is_empty(s)) {
printf("Stack is empty.\n");
return NULL;
}
return s->arr[s->top--];
}
//创建二叉树
TreeNode* create_tree() {
int data;
scanf("%d", &data);
if (data == -1) {
return NULL;
}
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->data = data;
root->left = create_tree();
root->right = create_tree();
return root;
}
//非递归中序遍历二叉树
void inorder_traversal(TreeNode* root) {
Stack s;
init(&s);
TreeNode* p = root;
while (p || !is_empty(&s)) {
if (p) {
push(&s, p);
p = p->left;
}
else {
p = pop(&s);
printf("%d ", p->data);
p = p->right;
}
}
}
int main() {
printf("Please input the preorder traversal of the binary tree(-1 represents null node):\n");
TreeNode* root = create_tree();
printf("The inorder traversal of the binary tree is:\n");
inorder_traversal(root);
printf("\n");
return 0;
}
```
以下是几个输入输出例子:
例子1:
输入:
```
Please input the preorder traversal of the binary tree(-1 represents null node):
1 2 -1 -1 3 -1 -1
```
输出:
```
The inorder traversal of the binary tree is:
2 1 3
```
例子2:
输入:
```
Please input the preorder traversal of the binary tree(-1 represents null node):
1 2 4 -1 -1 5 7 -1 -1 -1 3 -1 6 -1 -1
```
输出:
```
The inorder traversal of the binary tree is:
4 2 7 5 1 3 6
```
例子3:
输入:
```
Please input the preorder traversal of the binary tree(-1 represents null node):
1 2 4 -1 -1 -1 3 6 -1 -1 7 -1 -1
```
输出:
```
The inorder traversal of the binary tree is:
4 2 1 6 3 7
```
阅读全文
相关推荐
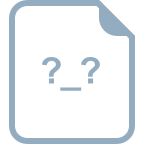






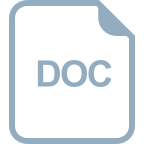








