用C++代码写出顺序表的排序、有序表的合并、集合的合并
时间: 2024-10-13 17:18:16 浏览: 26
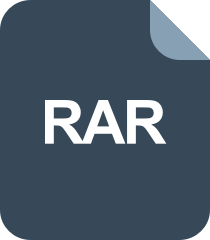
《数据结构—使用c++语言描述》 陈惠南 第二版 课后习题答案

在C++中,以下是针对不同类型数据结构的操作:
1. **顺序表排序** (假设我们有一个整数数组作为顺序表):
```cpp
#include <algorithm>
#include <vector>
// 假设arr是一个已声明的整数vector
void sortSequentialList(std::vector<int>& arr) {
std::sort(arr.begin(), arr.end());
}
```
这里使用了`std::sort()`函数,它是STL中的标准库算法,对数组进行升序排列。
2. **有序表合并** (两个升序排列的整数数组a和b):
```cpp
void mergeSortedArrays(int* a, int* b, int m, int n, int* result, int& resultSize) {
int i = 0, j = 0, k = 0;
while (i < m && j < n) {
if (a[i] <= b[j]) {
result[k++] = a[i++];
} else {
result[k++] = b[j++];
}
}
// 如果还有剩余元素,直接复制到结果数组
while (i < m) {
result[k++] = a[i++];
}
while (j < n) {
result[k++] = b[j++];
}
resultSize = k;
}
```
这个函数遍历两个输入数组,并将较小的元素依次放入结果数组。
3. **集合合并** (假设我们有两个包含唯一元素的`std::set`):
```cpp
void mergeSets(const std::set<int>& set1, const std::set<int>& set2, std::set<int>& mergedSet) {
for (const auto& elem : set2) {
mergedSet.insert(elem);
}
}
```
这里将第二个集合的所有元素插入到第一个集合中,由于`std::set`自动处理去重,所以不会有重复的元素。
阅读全文
相关推荐
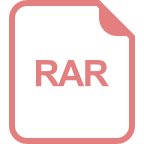
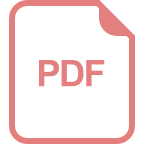
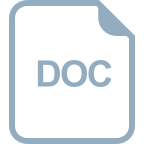
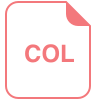
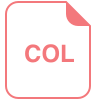
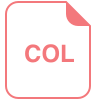
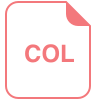
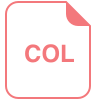
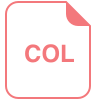
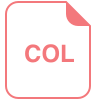
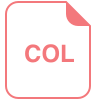
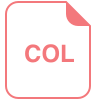
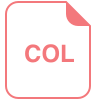
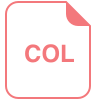
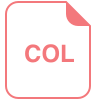
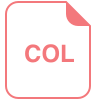
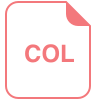