C++有向邻接表std::vector<std::vector<unsigned int>> graph,写一个函数,可以输出多个路径
时间: 2024-12-24 18:35:31 浏览: 4
在C++中,使用`std::vector<std::vector<unsigned int>>`表示有向邻接表,要输出从某个起点到终点的多个路径,我们可以创建一个深度优先搜索(DFS)或广度优先搜索(BFS)函数。这里我们以DFS为例:
```cpp
#include <iostream>
#include <vector>
void printPaths(const std::vector<std::vector<unsigned int>>& graph, unsigned int start, unsigned int end, std::vector<unsigned int>& path, bool found = false) {
if (start == end && found) {
// 如果到达终点并且已经找到路径,打印路径
std::cout << "Path: ";
for (auto& node : path) {
std::cout << node << " -> ";
}
std::cout << end << "\n";
return;
}
if (start == end) {
// 如果当前节点就是终点,但还没有找到路径,说明路径不存在
return;
}
// 探索当前节点的邻居
for (unsigned int neighbor : graph[start]) {
path.push_back(neighbor);
printPaths(graph, neighbor, end, path, true); // 递归调用并标记已找到路径
path.pop_back(); // 回溯,尝试下一个邻居
}
}
// 示例用法
int main() {
std::vector<std::vector<unsigned int>> graph = {{1, 2}, {2, 3}, {3, 4}, {4, 5}};
unsigned int start = 1, end = 5;
std::vector<unsigned int> path;
printPaths(graph, start, end, path);
return 0;
}
```
这个`printPaths`函数通过深度优先搜索遍历图,每次访问一个节点时,都会尝试将该节点添加到路径中,并继续向下探索直到找到终点。如果找到一条到达终点的路径,就打印出来。
阅读全文
相关推荐
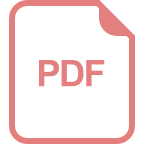
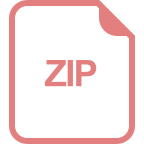
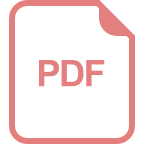



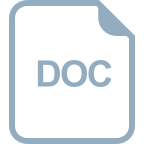
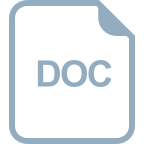
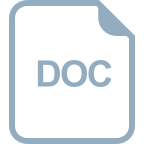
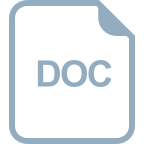
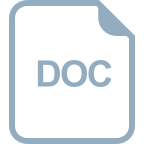







