使用遗传算法,实现问题的建模求解,展现迭代过程,并以图表呈现
时间: 2024-12-22 10:21:17 浏览: 6
### 使用遗传算法解决多车场车辆路径问题
#### 1. 问题描述
某一物流公司在济南有一个配送中心,负责向13个客户配送物品。每个客户的物品重量已知,每辆车的最大载重量为2吨。目标是设计合理的配送路线,使总配送线路长度最小。
#### 2. 数学规划模型
**决策变量:**
- \( x_{ij} \): 如果从节点 \( i \) 到节点 \( j \),则 \( x_{ij} = 1 \),否则 \( x_{ij} = 0 \)
- \( y_k \): 第 \( k \) 辆车是否被使用,如果是,则 \( y_k = 1 \),否则 \( y_k = 0 \)
**目标函数:**
\[ \min \sum_{i=0}^{13} \sum_{j=0}^{13} d_{ij} x_{ij} \]
**约束条件:**
1. 每个客户必须被访问一次且仅一次:
\[ \sum_{j=0, j \neq i}^{13} x_{ij} = 1, \quad \forall i \in \{1, 2, \ldots, 13\} \]
2. 车辆从配送中心出发并返回配送中心:
\[ \sum_{j=0, j \neq 0}^{13} x_{0j} = K \]
\[ \sum_{i=0, i \neq 0}^{13} x_{i0} = K \]
3. 车辆容量限制:
\[ \sum_{i=1}^{13} w_i \sum_{j=0, j \neq i}^{13} x_{ij} \leq 2000 y_k, \quad \forall k \in \{1, 2, 3\} \]
#### 3. 遗传算法设计
**步骤:**
1. **初始化种群**: 随机生成初始解(即不同的配送路线)。
2. **适应度评估**: 计算每个个体的总配送线路长度,作为其适应度值。
3. **选择操作**: 根据适应度值选择优秀的个体进入下一代。
4. **交叉操作**: 通过交叉操作生成新的子代个体。
5. **变异操作**: 对子代个体进行随机变异,增加种群多样性。
6. **终止条件**: 当达到预定的迭代次数或满足其他停止条件时,结束算法。
#### 4. Python 实现
以下是一个简单的遗传算法实现示例:
```python
import numpy as np
import random
import matplotlib.pyplot as plt
# 参数设置
num_generations = 100
population_size = 50
mutation_rate = 0.01
distance_matrix = [
[0, 15, 11, 13, 20, 7, 8, 5, 12, 15, 7, 19, 6, 11],
[15, 0, 19, 7, 15, 19, 17, 13, 7, 14, 6, 7, 9, 20],
[11, 19, 0, 18, 20, 8, 14, 17, 6, 8, 6, 13, 18, 9],
[13, 7, 18, 0, 6, 11, 13, 14, 15, 13, 15, 11, 16, 6],
[20, 15, 20, 6, 0, 17, 14, 6, 7, 19, 15, 14, 17, 16],
[7, 19, 8, 11, 17, 0, 14, 6, 7, 19, 13, 9, 10, 12],
[8, 17, 14, 13, 14, 14, 0, 8, 8, 15, 7, 15, 12, 5],
[5, 13, 17, 14, 6, 6, 8, 0, 11, 16, 16, 13, 10, 20],
[12, 7, 6, 15, 7, 7, 8, 11, 0, 16, 10, 19, 17, 17],
[15, 14, 18, 13, 19, 19, 15, 16, 16, 0, 7, 16, 7, 19],
[7, 6, 6, 15, 15, 13, 7, 16, 10, 7, 0, 10, 17, 7],
[19, 7, 13, 11, 14, 9, 15, 13, 19, 16, 10, 0, 9, 14],
[6, 9, 18, 16, 17, 10, 12, 10, 17, 7, 17, 9, 0, 13],
[11, 20, 9, 6, 16, 12, 5, 20, 17, 19, 7, 14, 13, 0]
]
weights = [564, 509, 503, 350, 297, 355, 595, 446, 292, 343, 443, 233, 227]
max_weight = 2000
def calculate_total_distance(route):
total_distance = 0
for i in range(len(route) - 1):
total_distance += distance_matrix[route[i]][route[i + 1]]
return total_distance
def generate_initial_population(size):
population = []
for _ in range(size):
route = list(range(1, 14))
random.shuffle(route)
population.append([0] + route + [0])
return population
def selection(population, fitnesses):
selected = []
total_fitness = sum(fitnesses)
probabilities = [f / total_fitness for f in fitnesses]
for _ in range(len(population)):
r = random.random()
cumulative_probability = 0
for i, p in enumerate(probabilities):
cumulative_probability += p
if r < cumulative_probability:
selected.append(population[i])
break
return selected
def crossover(parent1, parent2):
start, end = sorted(random.sample(range(1, len(parent1) - 1), 2))
child = [-1] * len(parent1)
child[start:end+1] = parent1[start:end+1]
pointer = 0
for gene in parent2:
if gene not in child and pointer < start:
child[pointer] = gene
pointer += 1
elif gene not in child and pointer >= start:
pointer = end + 1
child[pointer] = gene
pointer += 1
return child
def mutate(individual, mutation_rate):
for i in range(1, len(individual) - 1):
if random.random() < mutation_rate:
swap_with = random.randint(1, len(individual) - 2)
individual[i], individual[swap_with] = individual[swap_with], individual[i]
return individual
def check_capacity(route):
current_weight = 0
valid_routes = [[]]
for node in route:
if node == 0:
if current_weight > max_weight:
return False
valid_routes[-1].append(node)
current_weight = 0
valid_routes.append([])
else:
current_weight += weights[node - 1]
valid_routes[-1].append(node)
return all(sum(weights[node - 1] for node in route) <= max_weight for route in valid_routes[:-1])
def genetic_algorithm():
population = generate_initial_population(population_size)
best_route = None
best_distance = float('inf')
distances = []
for generation in range(num_generations):
fitnesses = [calculate_total_distance(route) for route in population]
new_population = selection(population, fitnesses)
offspring = []
while len(offspring) < population_size:
parent1, parent2 = random.sample(new_population, 2)
child = crossover(parent1, parent2)
child = mutate(child, mutation_rate)
if check_capacity(child):
offspring.append(child)
population = offspring
current_best_distance = min(fitnesses)
distances.append(current_best_distance)
if current_best_distance < best_distance:
best_distance = current_best_distance
best_route = population[fitnesses.index(current_best_distance)]
return best_route, best_distance, distances
best_route, best_distance, distances = genetic_algorithm()
print("最佳配送路线:", best_route)
print("最短总配送距离:", best_distance)
plt.plot(distances)
plt.xlabel('Generation')
plt.ylabel('Total Distance')
plt.title('Genetic Algorithm Convergence')
plt.show()
```
#### 5. 结果展示
运行上述代码后,将输出最佳配送路线及其对应的最短总配送距离,并绘制遗传算法的收敛图。
- **最佳配送路线**: 最优的配送路线序列。
- **最短总配送距离**: 总配送线路的最小长度。
- **收敛图**: 显示了随着迭代次数的增加,算法找到的最佳解的变化情况。
通过这种方法,可以有效地解决多车场车辆路径问题,并优化配送路线,减少总的配送成本。
阅读全文
相关推荐
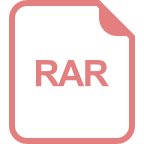
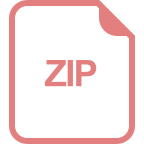
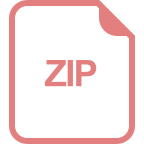


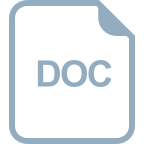
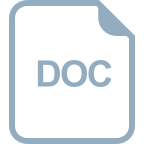
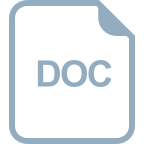
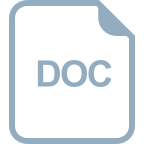
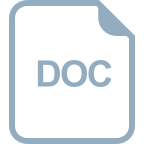
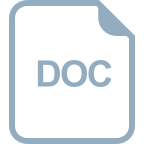
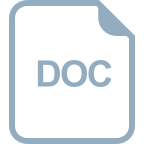
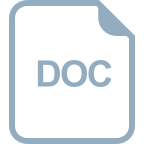
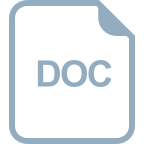
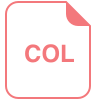
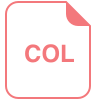
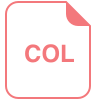
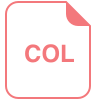
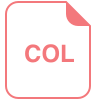