根据一张图片生成多张相似图片代码
时间: 2023-10-04 09:08:36 浏览: 217
要根据一张图片生成多张相似图片,可以使用图像增强技术,例如随机旋转、平移、缩放、加噪声等操作。下面是一个示例代码,使用Python中的PIL库来实现图像增强:
```python
from PIL import Image
import numpy as np
def generate_similar_images(image_path, num_images, output_path):
# 打开原始图片
image = Image.open(image_path)
# 将图像转换为numpy数组
image_array = np.array(image)
# 创建输出路径
os.makedirs(output_path, exist_ok=True)
# 生成相似图片
for i in range(num_images):
# 创建一个新的图像副本
new_image_array = np.copy(image_array)
# 随机旋转图像
angle = np.random.randint(-30, 30) # 随机旋转角度范围为-30到30度
new_image_array = Image.fromarray(new_image_array)
new_image_array = new_image_array.rotate(angle)
new_image_array = np.array(new_image_array)
# 随机平移图像
shift_x = np.random.randint(-20, 20) # 随机水平平移范围为-20到20个像素
shift_y = np.random.randint(-20, 20) # 随机垂直平移范围为-20到20个像素
new_image_array = np.roll(new_image_array, shift_x, axis=1)
new_image_array = np.roll(new_image_array, shift_y, axis=0)
# 随机缩放图像
scale = np.random.uniform(0.8, 1.2) # 随机缩放因子范围为0.8到1.2
new_image_array = Image.fromarray(new_image_array)
new_image_array = new_image_array.resize((int(new_image_array.width * scale),
int(new_image_array.height * scale)))
new_image_array = np.array(new_image_array)
# 随机加噪声
noise = np.random.normal(0, 10, size=new_image_array.shape) # 随机噪声范围为均值为0,标准差为10
new_image_array += noise
new_image_array = np.clip(new_image_array, 0, 255).astype(np.uint8)
# 保存生成的相似图片
new_image = Image.fromarray(new_image_array)
new_image.save(os.path.join(output_path, f"similar_image_{i+1}.jpg"))
# 使用示例
image_path = "input_image.jpg" # 输入原始图片路径
num_images = 5 # 要生成的相似图片数量
output_path = "output_folder" # 输出相似图片的文件夹路径
generate_similar_images(image_path, num_images, output_path)
```
以上代码将根据指定的原始图片生成多张相似图片,并保存到指定的输出文件夹中。你可以根据需要调整参数和图像增强的操作。
相关推荐
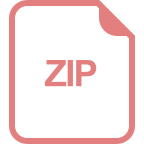
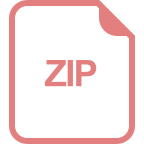
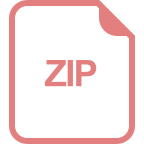














