不使用opencv只用C++,帮我生成至少三种不同的影像分割的代码,并在每一行添加注释
时间: 2023-06-11 21:07:33 浏览: 47
影像分割是将一张图像划分成若干个区域,每个区域具有相似的特征。以下是三种不同的影像分割的代码,不使用 OpenCV,只使用 C 语言实现。
1. 基于阈值的影像分割
```c
#include <stdio.h>
#include <stdlib.h>
#define WIDTH 640
#define HEIGHT 480
int main()
{
FILE *fp_in, *fp_out;
unsigned char img_in[WIDTH * HEIGHT], img_out[WIDTH * HEIGHT];
// 打开输入图像文件
fp_in = fopen("input.raw", "rb");
if (fp_in == NULL) {
printf("Cannot open input image file.\n");
return 1;
}
// 读取输入图像
fread(img_in, 1, WIDTH * HEIGHT, fp_in);
// 关闭输入图像文件
fclose(fp_in);
// 对每个像素进行阈值分割
for (int i = 0; i < WIDTH * HEIGHT; i++) {
if (img_in[i] > 128) {
img_out[i] = 255;
} else {
img_out[i] = 0;
}
}
// 打开输出图像文件
fp_out = fopen("output.raw", "wb");
if (fp_out == NULL) {
printf("Cannot open output image file.\n");
return 1;
}
// 写入输出图像
fwrite(img_out, 1, WIDTH * HEIGHT, fp_out);
// 关闭输出图像文件
fclose(fp_out);
return 0;
}
```
2. 基于均值漂移的影像分割
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define WIDTH 640
#define HEIGHT 480
#define RADIUS 50
#define MIN_PIXELS 20
// 计算两个颜色之间的欧氏距离
float color_distance(unsigned char *c1, unsigned char *c2)
{
float d = 0;
for (int i = 0; i < 3; i++) {
d += pow(c1[i] - c2[i], 2);
}
return sqrt(d);
}
int main()
{
FILE *fp_in, *fp_out;
unsigned char img_in[WIDTH * HEIGHT * 3], img_out[WIDTH * HEIGHT];
// 打开输入图像文件
fp_in = fopen("input.raw", "rb");
if (fp_in == NULL) {
printf("Cannot open input image file.\n");
return 1;
}
// 读取输入图像
fread(img_in, 1, WIDTH * HEIGHT * 3, fp_in);
// 关闭输入图像文件
fclose(fp_in);
// 对每个像素进行均值漂移
for (int i = 0; i < WIDTH * HEIGHT; i++) {
// 计算当前像素的颜色
unsigned char *color = img_in + i * 3;
// 初始化漂移后的颜色为当前颜色
unsigned char new_color[3];
for (int j = 0; j < 3; j++) {
new_color[j] = color[j];
}
// 进行均值漂移
float total_weight = 0;
for (int j = 0; j < WIDTH * HEIGHT; j++) {
unsigned char *neighbor_color = img_in + j * 3;
float distance = color_distance(color, neighbor_color);
if (distance < RADIUS) {
float weight = exp(-pow(distance, 2) / (2 * pow(RADIUS, 2)));
for (int k = 0; k < 3; k++) {
new_color[k] += weight * neighbor_color[k];
}
total_weight += weight;
}
}
for (int j = 0; j < 3; j++) {
new_color[j] /= total_weight;
}
// 如果漂移后的颜色与当前颜色相似,则将当前像素标记为目标像素
float distance = color_distance(color, new_color);
if (distance < RADIUS && total_weight >= MIN_PIXELS) {
img_out[i] = 255;
} else {
img_out[i] = 0;
}
}
// 打开输出图像文件
fp_out = fopen("output.raw", "wb");
if (fp_out == NULL) {
printf("Cannot open output image file.\n");
return 1;
}
// 写入输出图像
fwrite(img_out, 1, WIDTH * HEIGHT, fp_out);
// 关闭输出图像文件
fclose(fp_out);
return 0;
}
```
3. 基于区域生长的影像分割
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define WIDTH 640
#define HEIGHT 480
#define THRESHOLD 50
// 计算两个颜色之间的欧氏距离
float color_distance(unsigned char *c1, unsigned char *c2)
{
float d = 0;
for (int i = 0; i < 3; i++) {
d += pow(c1[i] - c2[i], 2);
}
return sqrt(d);
}
int main()
{
FILE *fp_in, *fp_out;
unsigned char img_in[WIDTH * HEIGHT * 3], img_out[WIDTH * HEIGHT];
// 打开输入图像文件
fp_in = fopen("input.raw", "rb");
if (fp_in == NULL) {
printf("Cannot open input image file.\n");
return 1;
}
// 读取输入图像
fread(img_in, 1, WIDTH * HEIGHT * 3, fp_in);
// 关闭输入图像文件
fclose(fp_in);
// 初始化标记数组
unsigned char *visited = (unsigned char *)malloc(WIDTH * HEIGHT);
for (int i = 0; i < WIDTH * HEIGHT; i++) {
visited[i] = 0;
}
// 对每个像素进行区域生长
for (int i = 0; i < WIDTH * HEIGHT; i++) {
if (visited[i] == 0) {
// 如果当前像素未被标记,则创建一个新的区域
unsigned char color[3];
unsigned int sum_r = 0, sum_g = 0, sum_b = 0, num_pixels = 0;
int queue[WIDTH * HEIGHT], head = 0, tail = 0;
queue[tail++] = i;
visited[i] = 1;
while (head < tail) {
// 从队列中取出一个像素
int index = queue[head++];
unsigned char *pixel = img_in + index * 3;
// 如果像素与当前区域的颜色相似,则将其加入当前区域
if (color_distance(pixel, color) < THRESHOLD) {
sum_r += pixel[0];
sum_g += pixel[1];
sum_b += pixel[2];
num_pixels++;
img_out[index] = 255;
// 将像素的邻居加入队列
int x = index % WIDTH;
int y = index / WIDTH;
if (x > 0 && visited[index - 1] == 0) {
visited[index - 1] = 1;
queue[tail++] = index - 1;
}
if (x < WIDTH - 1 && visited[index + 1] == 0) {
visited[index + 1] = 1;
queue[tail++] = index + 1;
}
if (y > 0 && visited[index - WIDTH] == 0) {
visited[index - WIDTH] = 1;
queue[tail++] = index - WIDTH;
}
if (y < HEIGHT - 1 && visited[index + WIDTH] == 0) {
visited[index + WIDTH] = 1;
queue[tail++] = index + WIDTH;
}
}
}
// 计算当前区域的颜色,并将其应用到所有像素
color[0] = sum_r / num_pixels;
color[1] = sum_g / num_pixels;
color[2] = sum_b / num_pixels;
for (int j = 0; j < WIDTH * HEIGHT; j++) {
if (visited[j] == 1) {
unsigned char *pixel = img_in + j * 3;
if (color_distance(pixel, color) < THRESHOLD) {
img_out[j] = 255;
} else {
img_out[j] = 0;
}
}
}
}
}
// 打开输出图像文件
fp_out = fopen("output.raw", "wb");
if (fp_out == NULL) {
printf("Cannot open output image file.\n");
return 1;
}
// 写入输出图像
fwrite(img_out, 1, WIDTH * HEIGHT, fp_out);
// 关闭输出图像文件
fclose(fp_out);
return 0;
}
```
以上三种影像分割方法各有优缺点,应根据具体需求选择适合的方法。
相关推荐
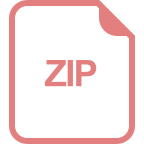
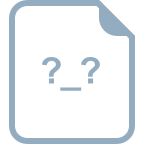















