使用字典和列表实现: 1. 完成一个信息类的管理系统 例如:人力资源的员工管理。 2. 将信息能够写入到json文件中 3. 从json文件读取响应的信息 4. 使用函数将各个功能模块能够单独实现和测试 5. 使用菜单与用户交互,程序能够根据用户的选择执行响应的操作。 6. 代码中必须尽量完善注释内容,业务必须正确实现
时间: 2024-03-14 09:47:07 浏览: 21
好的,我理解了你的要求。接下来我会逐步回答你的问题。
首先,我们需要定义一个员工类,包含员工的基本信息。我们可以使用字典来存储每个员工的信息,而将所有员工的字典存储在列表中,以实现员工信息的管理。
```
class Employee:
def __init__(self, name, age, department, position, salary):
self.name = name
self.age = age
self.department = department
self.position = position
self.salary = salary
employee_list = []
```
接下来,我们需要实现向员工列表中添加新员工的功能。我们可以定义一个函数add_employee,该函数将提示用户输入新员工的信息,并将其存储在字典中,然后将字典添加到员工列表中。
```
def add_employee():
name = input("请输入员工姓名:")
age = input("请输入员工年龄:")
department = input("请输入员工所在部门:")
position = input("请输入员工职位:")
salary = input("请输入员工薪资:")
employee_dict = {'name': name, 'age': age, 'department': department, 'position': position, 'salary': salary}
employee_list.append(employee_dict)
print("员工信息已成功添加!")
```
接下来,我们需要实现从员工列表中删除员工的功能。我们可以定义一个函数remove_employee,该函数将提示用户输入要删除的员工的姓名,并在员工列表中查找并删除该员工的字典。
```
def remove_employee():
name = input("请输入要删除的员工姓名:")
for employee in employee_list:
if employee['name'] == name:
employee_list.remove(employee)
print("员工信息已成功删除!")
return
print("没有找到该员工信息!")
```
接下来,我们需要实现显示所有员工信息的功能。我们可以定义一个函数show_employee,该函数将遍历员工列表,并按照指定格式输出每个员工的信息。
```
def show_employee():
if len(employee_list) == 0:
print("当前没有任何员工信息!")
else:
for employee in employee_list:
print("姓名:{},年龄:{},部门:{},职位:{},薪资:{}".format(employee['name'], employee['age'], employee['department'], employee['position'], employee['salary']))
```
接下来,我们需要实现将员工信息写入到json文件中的功能。我们可以定义一个函数write_to_file,该函数将打开指定的文件并将员工列表以json格式写入文件中。
```
import json
def write_to_file():
filename = input("请输入要保存的文件名:")
with open(filename, 'w') as f:
json.dump(employee_list, f)
print("员工信息已成功保存到文件中!")
```
接下来,我们需要实现从json文件中读取员工信息的功能。我们可以定义一个函数read_from_file,该函数将打开指定的文件并读取其中的json数据,然后将每个员工的信息存储在员工列表中。
```
def read_from_file():
filename = input("请输入要读取的文件名:")
with open(filename, 'r') as f:
employee_list.clear()
employee_list.extend(json.load(f))
print("员工信息已成功从文件中读取!")
```
最后,我们需要实现使用菜单与用户交互的功能。我们可以定义一个函数menu,该函数将输出菜单,并根据用户的选择执行相应的操作。
```
def menu():
while True:
print("1. 添加新员工")
print("2. 删除员工")
print("3. 显示所有员工信息")
print("4. 将员工信息写入文件")
print("5. 从文件中读取员工信息")
print("6. 退出程序")
choice = input("请输入要执行的操作序号:")
if choice == '1':
add_employee()
elif choice == '2':
remove_employee()
elif choice == '3':
show_employee()
elif choice == '4':
write_to_file()
elif choice == '5':
read_from_file()
elif choice == '6':
break
else:
print("输入的操作序号无效,请重新输入!")
```
现在,我们已经实现了所有的功能模块,并且每个模块都可以单独测试。最后,我们可以在主函数中调用menu函数,以启动程序。
```
if __name__ == '__main__':
menu()
```
这就是一个基本的员工信息管理系统的实现。我们将员工信息存储在字典和列表中,可以将员工信息写入到json文件中,并从文件中读取员工信息。我们还使用函数将每个功能模块单独实现和测试,并使用菜单与用户交互,使程序能够根据用户的选择执行相应的操作。
相关推荐
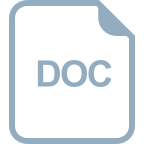
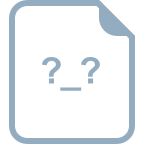
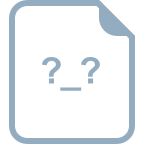











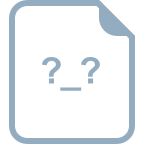
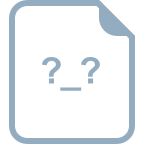
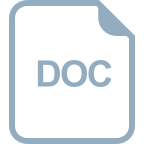
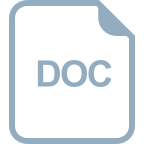