本实验要求通过MapReduce程序实现简单的数据统计,输入文件是一组文本文件,每个输入文件中都包含很多行,每行都是一个数字字符串,代表了一个特别大的数字。编写一个程序求包含在输入文件中的所有数字的和、最大值、最小值、平均值并输出
时间: 2024-05-20 08:19:24 浏览: 92
好的,这个问题可以通过以下步骤来解决:
1.分析问题:我们需要对一组文本文件进行数据统计,包括求和、最大值、最小值、平均值,这些数据统计需要在多个文本文件中进行,并且每个文件可能会包含很多行数据。考虑到这些数据可能非常大,我们需要使用MapReduce程序来并行处理这些数据。
2.设计MapReduce程序:我们可以设计一个MapReduce程序来实现这个功能。Map函数将每个输入文件中的每一行数据读入,将其转换为数字,并将其作为键值对传递给Reduce函数。Reduce函数将所有的数字进行累加、求最大值、最小值、平均值等操作,最终输出统计结果。
3.实现MapReduce程序:我们可以使用Hadoop或者其他MapReduce框架来实现这个程序。具体实现过程中,我们需要实现Map函数和Reduce函数,并将其打包成Jar包上传到Hadoop集群上运行。
4.测试MapReduce程序:我们可以使用一些测试数据来验证程序的正确性。例如,我们可以创建一组包含很多数字的文本文件,并使用MapReduce程序来统计这些数字的和、最大值、最小值、平均值等数据。如果程序能够正确输出这些数据,那么就说明程序实现正确。
总结:通过以上步骤,我们可以使用MapReduce程序来实现对一组文本文件中的数字进行数据统计。这种方法可以有效地处理大规模数据,并且具有良好的可扩展性和容错性。
相关问题
通过编程HadoopMapReduce程序实现简单的数据统计,输入文件是一组文本文件,每个输入文件中都包含很多行,每行都是一个数字字符串,代表了一个特别大的数字。编写一个程序求包含在输入文件中的所有数字的和、最大值、最小值、平均值并输出
以下是一个使用Hadoop MapReduce框架的实现:
首先,定义Mapper类和Reducer类。Mapper类的作用是将每个数字字符串转换为一个数字对,并将其发送到Reducer类。Reducer类的作用是计算输入数字的和、最大值、最小值和计数,并在最后输出结果。
```java
import java.io.IOException;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.*;
import org.apache.hadoop.mapreduce.*;
public class SimpleStatistics {
public static class TokenizerMapper extends Mapper<Object, Text, NullWritable, IntWritable>{
private final static IntWritable one = new IntWritable(1);
private IntWritable number = new IntWritable();
public void map(Object key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
String[] fields = line.split(",");
for (String field : fields) {
try {
int num = Integer.parseInt(field.trim());
number.set(num);
context.write(NullWritable.get(), number);
} catch (NumberFormatException e) {
// ignore invalid numbers
}
}
}
}
public static class IntSumReducer extends Reducer<NullWritable,IntWritable,Text,IntWritable> {
private IntWritable result = new IntWritable();
private Text type = new Text();
public void reduce(NullWritable key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
int count = 0;
int max = Integer.MIN_VALUE;
int min = Integer.MAX_VALUE;
for (IntWritable val : values) {
int num = val.get();
sum += num;
count++;
if (num > max) {
max = num;
}
if (num < min) {
min = num;
}
}
if (count > 0) {
result.set(sum);
type.set("sum");
context.write(type, result);
result.set(max);
type.set("max");
context.write(type, result);
result.set(min);
type.set("min");
context.write(type, result);
result.set(sum / count);
type.set("avg");
context.write(type, result);
}
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "simple statistics");
job.setJarByClass(SimpleStatistics.class);
job.setMapperClass(TokenizerMapper.class);
job.setCombinerClass(IntSumReducer.class);
job.setReducerClass(IntSumReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
for (int i = 0; i < args.length - 1; i++) {
Path inputPath = new Path(args[i]);
job.addInputPath(inputPath);
}
Path outputPath = new Path(args[args.length - 1]);
job.setOutputPath(outputPath);
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
在上述代码中,Mapper类的map()函数将输入的数字字符串转换为IntWritable类型,并将其写入上下文。Reducer类的reduce()函数计算输入数字的和、最大值、最小值和计数,并将结果输出到上下文。
在main()函数中,我们设置了Mapper类和Reducer类,指定输入和输出路径,并运行Job。运行程序时,使用以下命令:
```shell
hadoop jar SimpleStatistics.jar input1,input2,..,inputN output
```
其中,input1,input2,..,inputN是输入文件的路径,用逗号分隔。output是输出文件的路径。
执行后,输出文件将包含以下统计数据:
- sum:所有数字的总和
- max:最大值
- min:最小值
- avg:平均值
希望这能帮到你!
阅读全文
相关推荐
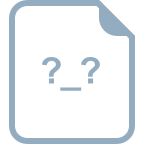
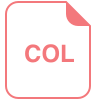
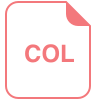
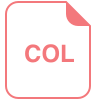
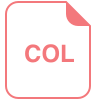
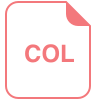
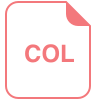
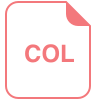
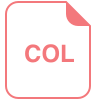
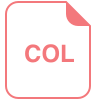
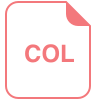
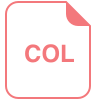
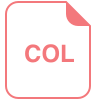
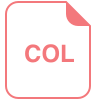
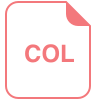