如何实现一个函数同时用于检查字符串是否为回文以及校验括号是否匹配,用c语言
时间: 2024-12-02 16:14:33 浏览: 16
在C语言中,我们可以创建一个函数,该函数接收一个字符串作为输入,并通过两个嵌套的条件判断来完成回文检查和括号匹配的验证。以下是函数的基本实现:
```c
#include <stdio.h>
#include <stdbool.h>
#include <ctype.h>
// 函数用来检查字符串是否是回文
bool is_palindrome(const char* str) {
int start = 0;
int end = strlen(str) - 1;
while (start < end) {
if (str[start] != str[end]) {
return false;
}
start++;
end--;
}
return true;
}
// 函数用来检查括号是否匹配
bool are_parens_matched(const char* str) {
stack<char> stack; // 使用栈来辅助判断
for (const char c : str) {
if (c == '(' || c == '{' || c == '[') { // 入栈非空元素
stack.push(c);
} else if (c == ')' || c == '}' || c == ']') { // 遇到闭合符
if (stack.empty()) {
return false; // 栈为空,直接返回不匹配
}
char top = stack.top();
stack.pop();
if ((c == ')' && top != '(') ||
(c == '}' && top != '{') ||
(c == ']' && top != '[')) {
return false; // 匹配失败
}
}
}
return stack.empty(); // 如果所有打开的括号都已关闭,则返回true
}
// 主函数
int main() {
const char* input = "aba(){}[]";
printf("Is the string a palindrome? %s\n", is_palindrome(input) ? "Yes" : "No");
printf("Are the parentheses matched? %s\n", are_parens_matched(input) ? "Yes" : "No");
return 0;
}
```
阅读全文
相关推荐
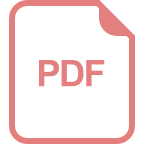
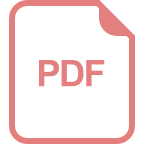
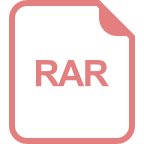















