如何在C语言中实现一个函数来检查一个字符串是否是回文串?
时间: 2024-12-19 12:15:52 浏览: 9
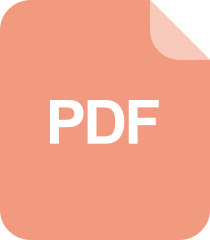
回文字符串:判断一个是否是回文字符串。回文字符串是指正序(从左向右)和倒序(从右向左)读都是一样的字符串。

在C语言中,要实现一个函数来检查一个字符串是否是回文串,你可以创建一个名为`isPalindrome`的函数,它接受一个指向字符数组的指针,并通过比较字符串的第一个和最后一个字符、第二个和倒数第二个字符,直到中间位置,判断它们是否相等。如果整个过程所有对应的字符都匹配,则该字符串是回文。
下面是一个简单的示例:
```c
#include <stdbool.h>
#include <string.h>
// 函数声明
bool isPalindrome(const char* str);
// 实现
bool isPalindrome(const char* str) {
int start = 0;
int end = strlen(str) - 1; // 获取字符串长度
while (start < end) { // 只需比较到字符串中心
if (str[start] != str[end]) {
return false; // 如果任意一对对应字符不相等,立即返回false
}
start++;
end--;
}
return true; // 所有字符匹配,说明是回文
}
int main() {
const char* testStr1 = "madam";
const char* testStr2 = "hello";
printf("%s is a palindrome? %d\n", testStr1, isPalindrome(testStr1));
printf("%s is a palindrome? %d\n", testStr2, isPalindrome(testStr2));
return 0;
}
```
在这个例子中,`isPalindrome`函数会分别检查`testStr1`和`testStr2`,并输出结果。
阅读全文
相关推荐
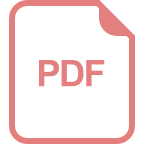
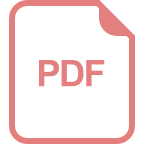















