java贪吃蛇源代码
时间: 2023-11-24 20:51:23 浏览: 42
以下是Java贪吃蛇的源代码,包括注释,希望对你有所帮助:
```java
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Snake extends JPanel implements KeyListener, Runnable {
private static final long serialVersionUID = 1L;
// 游戏区域的宽度和高度
private static final int WIDTH = 800;
private static final int HEIGHT = 600;
// 蛇的初始长度
private static final int INIT_LENGTH = 3;
// 蛇的每个方块的大小
private static final int BLOCK_SIZE = 20;
// 游戏区域的行数和列数
private static final int ROWS = HEIGHT / BLOCK_SIZE;
private static final int COLS = WIDTH / BLOCK_SIZE;
// 蛇的移动速度
private static final int SPEED = 100;
// 游戏区域
private Point[][] area = new Point[ROWS][COLS];
// 蛇的身体
private Point[] body = new Point[ROWS * COLS];
// 蛇的长度
private int length = INIT_LENGTH;
// 蛇的方向
private int direction = KeyEvent.VK_RIGHT;
// 食物的位置
private Point food = new Point();
// 游戏是否结束
private boolean gameOver = false;
// 游戏是否暂停
private boolean paused = false;
// 游戏得分
private int score = 0;
// 随机数生成器
private Random random = new Random();
// 构造函数
public Snake() {
// 初始化游戏区域
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
area[i][j] = new Point(j * BLOCK_SIZE, i * BLOCK_SIZE);
}
}
// 初始化蛇的身体
for (int i = 0; i < INIT_LENGTH; i++) {
body[i] = new Point(COLS / 2 - i, ROWS / 2);
}
// 初始化食物的位置
generateFood();
}
// 生成食物的位置
private void generateFood() {
int x = random.nextInt(COLS);
int y = random.nextInt(ROWS);
// 如果食物的位置和蛇的身体重合,则重新生成食物的位置
while (area[y][x].equals(food) || contains(body, length, new Point(x, y))) {
x = random.nextInt(COLS);
y = random.nextInt(ROWS);
}
food.setLocation(x, y);
}
// 判断点是否在数组中
private boolean contains(Point[] array, int length, Point point) {
for (int i = 0; i < length; i++) {
if (array[i].equals(point)) {
return true;
}
}
return false;
}
// 绘制游戏区域
private void drawArea(Graphics g) {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
g.setColor(Color.WHITE);
g.drawRect(area[i][j].x, area[i][j].y, BLOCK_SIZE, BLOCK_SIZE);
}
}
}
// 绘制蛇的身体
private void drawBody(Graphics g) {
for (int i = 0; i < length; i++) {
g.setColor(Color.GREEN);
g.fillRect(body[i].x * BLOCK_SIZE, body[i].y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
}
// 绘制食物
private void drawFood(Graphics g) {
g.setColor(Color.RED);
g.fillOval(food.x * BLOCK_SIZE, food.y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
// 绘制得分
private void drawScore(Graphics g) {
g.setColor(Color.BLACK);
g.setFont(new Font("宋体", Font.BOLD, 20));
g.drawString("得分:" + score, 20, 30);
}
// 绘制游戏结束
private void drawGameOver(Graphics g) {
g.setColor(Color.BLACK);
g.setFont(new Font("宋体", Font.BOLD, 40));
g.drawString("游戏结束!", WIDTH / 2 - 120, HEIGHT / 2);
}
// 绘制游戏暂停
private void drawPaused(Graphics g) {
g.setColor(Color.BLACK);
g.setFont(new Font("宋体", Font.BOLD, 40));
g.drawString("游戏暂停!", WIDTH / 2 - 120, HEIGHT / 2);
}
// 绘制界面
private void draw(Graphics g) {
drawArea(g);
drawBody(g);
drawFood(g);
drawScore(g);
if (gameOver) {
drawGameOver(g);
}
if (paused) {
drawPaused(g);
}
}
// 移动蛇的身体
private void move() {
// 记录蛇尾的位置
Point tail = body[length - 1];
// 将蛇的身体向前移动一格
for (int i = length - 1; i > 0; i--) {
body[i] = body[i - 1];
}
// 根据方向移动蛇头
switch (direction) {
case KeyEvent.VK_UP:
body[0] = new Point(body[0].x, body[0].y - 1);
break;
case KeyEvent.VK_DOWN:
body[0] = new Point(body[0].x, body[0].y + 1);
break;
case KeyEvent.VK_LEFT:
body[0] = new Point(body[0].x - 1, body[0].y);
break;
case KeyEvent.VK_RIGHT:
body[0] = new Point(body[0].x + 1, body[0].y);
break;
}
// 如果蛇头和食物重合,则蛇的长度加1,得分加10,并重新生成食物的位置
if (body[0].equals(food)) {
body[length++] = tail;
score += 10;
generateFood();
}
// 如果蛇头撞到游戏区域外或者撞到自己的身体,则游戏结束
if (body[0].x < 0 || body[0].x >= COLS || body[0].y < 0 || body[0].y >= ROWS
|| contains(body, length - 1, body[0])) {
gameOver = true;
}
}
// 处理键盘事件
@Override
public void keyPressed(KeyEvent e) {
int key = e.getKeyCode();
// 如果按下空格键,则暂停或继续游戏
if (key == KeyEvent.VK_SPACE) {
paused = !paused;
}
// 如果游戏结束或暂停,则不处理键盘事件
if (gameOver || paused) {
return;
}
// 如果按下方向键,则改变蛇的方向
switch (key) {
case KeyEvent.VK_UP:
case KeyEvent.VK_DOWN:
case KeyEvent.VK_LEFT:
case KeyEvent.VK_RIGHT:
if (Math.abs(key - direction) != 2) {
direction = key;
}
break;
}
}
@Override
public void keyReleased(KeyEvent e) {
}
@Override
public void keyTyped(KeyEvent e) {
}
// 游戏循环
@Override
public void run() {
while (true) {
try {
Thread.sleep(SPEED);
} catch (InterruptedException e) {
e.printStackTrace();
}
// 如果游戏结束或暂停,则不移动蛇的身体
if (gameOver || paused) {
continue;
}
move();
repaint();
}
}
// 创建窗口并启动游戏
public void myJFrame() {
JFrame frame = new JFrame("贪吃蛇");
frame.setSize(WIDTH, HEIGHT);
frame.setLocationRelativeTo(null);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setResizable(false);
frame.addKeyListener(this);
frame.add(this);
frame.setVisible(true);
new Thread(this).start();
}
public static void main(String[] args) {
Snake snake = new Snake();
snake.myJFrame();
}
}
```
相关推荐
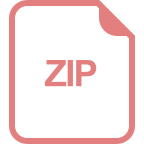
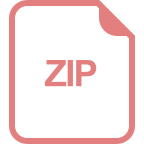










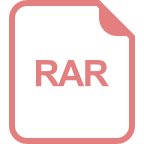