50行java小游戏源代码
时间: 2023-10-05 09:04:19 浏览: 180
以下是一个简单的 Java 小游戏代码,可以帮助您入门游戏开发。
```java
import java.awt.Color;
import java.awt.Graphics;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Game extends JPanel implements KeyListener {
private static final long serialVersionUID = 1L;
private int x = 0;
private int y = 0;
private int score = 0;
private int speed = 5;
private int blockSize = 10;
private int width = 400;
private int height = 400;
private boolean gameOver = false;
private Random random = new Random();
private int[] dx = {0, 0, -1, 1};
private int[] dy = {-1, 1, 0, 0};
private int direction = 1;
private int foodX = random.nextInt(width / blockSize) * blockSize;
private int foodY = random.nextInt(height / blockSize) * blockSize;
public Game() {
JFrame frame = new JFrame("Snake Game");
frame.setSize(width, height);
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.addKeyListener(this);
frame.add(this);
setFocusable(true);
setFocusTraversalKeysEnabled(false);
}
public void paint(Graphics g) {
super.paint(g);
if (gameOver) {
g.setColor(Color.RED);
g.drawString("Game Over", width / 2 - 30, height / 2);
g.drawString("Score: " + score, width / 2 - 30, height / 2 + 20);
return;
}
g.setColor(Color.BLACK);
g.fillRect(x, y, blockSize, blockSize);
g.setColor(Color.GREEN);
g.fillRect(foodX, foodY, blockSize, blockSize);
}
public void keyPressed(KeyEvent e) {
int keyCode = e.getKeyCode();
if (keyCode == KeyEvent.VK_UP && direction != 1) {
direction = 0;
}
if (keyCode == KeyEvent.VK_DOWN && direction != 0) {
direction = 1;
}
if (keyCode == KeyEvent.VK_LEFT && direction != 3) {
direction = 2;
}
if (keyCode == KeyEvent.VK_RIGHT && direction != 2) {
direction = 3;
}
}
public void keyReleased(KeyEvent e) {
}
public void keyTyped(KeyEvent e) {
}
public void move() {
x += dx[direction] * blockSize;
y += dy[direction] * blockSize;
if (x < 0 || x >= width || y < 0 || y >= height) {
gameOver = true;
}
if (x == foodX && y == foodY) {
score++;
foodX = random.nextInt(width / blockSize) * blockSize;
foodY = random.nextInt(height / blockSize) * blockSize;
}
}
public void run() {
while (!gameOver) {
move();
repaint();
try {
Thread.sleep(speed);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
Game game = new Game();
game.run();
}
}
```
这个游戏是一个简单的贪吃蛇游戏,玩家通过控制蛇的方向来吃到食物,每吃一个食物得分一分,游戏结束条件是撞墙或者撞到自己的身体。
阅读全文
相关推荐
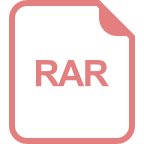
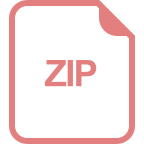
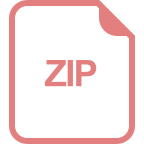















