写一个层次聚类谱系图的代码 python实现
时间: 2023-12-01 22:05:09 浏览: 34
以下是一个简单的 Python 代码实现层次聚类谱系图:
```python
import matplotlib.pyplot as plt
from scipy.cluster.hierarchy import dendrogram, linkage
# 数据准备,这里数据包含10个点,每个点有两个维度
X = [[0, 0], [0.1, 0], [1,1], [2,2],[2.1,2.1], [3,3], [10,10], [10,11], [11,10], [11,11]]
# 对 X 数据进行层次聚类,使用最简单的欧几里得距离度量和比较任务
Z = linkage(X, 'ward')
# 用 dendrogram 函数画图,可以看到分层聚类的谱系图
plt.figure(figsize=(10, 5))
dendrogram(Z)
plt.show()
```
这段代码使用了 scipy 包中的 `linkage()` 函数对数据进行层次聚类,该函数返回对X数据的一系列合并操作,其中 ward 参数选择了最小化各类内方差平方和的平方和来进行优化的方法,可以根据不同的应用场景选择不同的方法。最后,使用 matplotlib 的 `dendrogram()` 函数画出用于层次聚类的谱系图。
相关问题
请用Python实现层次聚类,并绘制谱系图
好的,我可以为您提供一个用Python实现层次聚类的示例代码,并使用matplotlib库绘制谱系图。请先安装scipy和matplotlib库:
```
pip install scipy
pip install matplotlib
```
接下来是示例代码:
```python
import scipy.cluster.hierarchy as shc
import numpy as np
import matplotlib.pyplot as plt
# 生成随机数据
X = np.random.rand(10, 2)
# 计算距离矩阵
distance_matrix = shc.distance.pdist(X)
# 计算层次聚类结果
linkage = shc.linkage(distance_matrix, method='ward')
# 绘制谱系图
plt.figure(figsize=(10, 7))
plt.title("Dendrogram")
dend = shc.dendrogram(linkage)
plt.show()
```
运行后,您将看到生成的谱系图。您可以将X替换为您的数据,以便进行层次聚类和绘制谱系图。
注意:这只是一个示例代码,您需要根据您的实际需求进行适当的修改和调整。
请用Python实现对导演进行层次聚类,并绘制谱系图
好的,我可以帮你解决这个问题。为了实现导演的层次聚类,我们需要使用Python中的SciPy库。具体步骤如下:
1. 收集导演的数据,可以从IMDb或其他电影数据库中获取。
2. 对导演数据进行处理,转换成适合聚类的形式。在这个例子中,我们可以使用导演的电影作为特征,创建一个电影-导演矩阵。
3. 使用SciPy库中的层次聚类函数对导演进行聚类。在这个例子中,我们可以使用Ward算法进行聚类。
4. 绘制聚类谱系图。可以使用SciPy库中的dendrogram函数来实现。
下面是一份Python代码示例,可以帮助你更好地理解这个过程:
```python
import numpy as np
import pandas as pd
from scipy.cluster.hierarchy import dendrogram, linkage
import matplotlib.pyplot as plt
# 读取导演数据
directors_data = pd.read_csv('directors.csv')
# 创建电影-导演矩阵
directors_movies = directors_data.pivot_table(index='director_name', columns='movie_title', values='movie_title', aggfunc='count')
directors_movies = directors_movies.fillna(0)
directors_movies = directors_movies.T
# 计算导演之间的距离
dist = linkage(directors_movies, 'ward')
# 绘制聚类谱系图
fig, ax = plt.subplots(figsize=(15, 20))
ax = dendrogram(dist, labels=directors_movies.index, orientation='left')
plt.tick_params(axis='both', which='both', bottom=False, top=False, labelbottom=False, right=False, left=False, labelleft=True)
plt.tight_layout()
plt.show()
```
在上面的代码中,我们假设导演数据保存在一个名为“directors.csv”的文件中。你需要根据自己的情况进行相应的修改。运行这段代码后,你将得到一个聚类谱系图,用于展示导演之间的聚类关系。
相关推荐










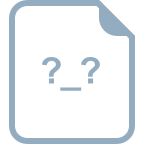
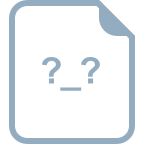