python辨别性别
时间: 2024-12-26 20:14:48 浏览: 7
### 使用 Python 实现性别辨别的方法
#### 方法一:基于预训练模型的面部特征分析
通过加载预先训练好的卷积神经网络(CNN),可以对输入的人脸图片进行分类预测。此过程涉及图像预处理、模型加载和最终预测三个主要部分。
```python
import numpy as np
from keras.models import load_model
import cv2
# 加载已经训练完成的性别分类器模型
gender_classifier = load_model('path_to_gender_model.h5')
def predict_gender(image_path):
# 读取并调整大小到指定尺寸 (64x64像素)
face_image = cv2.imread(image_path, 0)
resized_img = cv2.resize(face_image, (64, 64))
# 归一化处理并将数据转换成适合CNN输入的形式
normalized_resized_img = resized_img / 255.0
reshaped_normalized_resized_img = np.reshape(normalized_resized_img, (-1, 64, 64, 1))
# 预测性别标签索引
gender_label_arg = np.argmax(gender_classifier.predict(reshaped_normalized_resized_img)[^1])
return ['Male', 'Female'][gender_label_arg]
print(predict_gender('face.jpg'))
```
这段代码展示了如何利用Keras库中的`load_model()`函数来导入之前保存下来的性别识别模型,并调用该模型来进行新样本的预测。注意这里假设使用的模型期望接收形状为`(None, 64, 64, 1)`的数据作为输入[^2];因此,在实际应用前可能还需要根据具体情况进行适当修改以匹配所选模型的要求。
#### 方法二:OCR技术解析身份证件信息
另一种方式是从含有个人身份信息的照片中提取文字内容,进而依据特定规则推断出持证人性别。这通常适用于中国居民二代身份证等官方证件上的固定格式文本字段。
```python
try:
from PIL import Image
except ImportError:
import image
import pytesseract
def get_idcard_info(img_file):
text = str(pytesseract.image_to_string(Image.open(img_file), lang='chi_sim')) # 中文模式下识别
lines = [line.strip() for line in text.split('\n') if line != ""]
sex_index = None
for i,line in enumerate(lines):
if "男" in line or "女" in line:
sex_index = i
if not sex_index:
raise ValueError("无法找到性别信息")
return {'sex':lines[sex_index].replace(" ", "")}
result=get_idcard_info('./id_card_example.jpeg')
print(result['sex'])
```
上述脚本运用了Tesseract OCR引擎配合PIL/Pillow库实现了对中国大陆地区第二代居民身份证正面照片的文字识别功能[^3]。对于其他类型的文档,则需相应调整参数设置或采用不同的模板匹配策略。
#### 方法三:基于UI自动化工具Airtest Studio
如果目标场景是在移动应用程序界面内操作,比如登录页面填写表单时自动填充性别选项,那么借助于图形匹配算法也可以间接达到目的。
```python
from airtest.core.api import *
init_device(platform="Android")
if exists(Template(r"./templates/tpl_male_button.png", threshold=0.8)):
touch(Template(r"./templates/tpl_male_button.png"))
elif exists(Template(r"./templates/tpl_female_button.png")):
touch(Template(r"./templates/tpl_female_button.png"))
sleep(2)
snapshot(filename="./screenshot_after_selection.png")
```
在这个例子中,程序会尝试查找界面上是否存在男性按钮(`tpl_male_button.png`)或者女性按钮(`tpl_female_button.png`)的截图模板文件,一旦发现就点击对应位置模拟用户交互行为[^5]。这种方法依赖于视觉相似度比较,所以准确性取决于提供的模板质量及其与真实环境的一致程度。
阅读全文
相关推荐
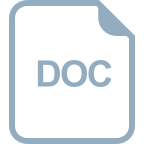
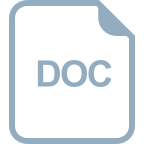
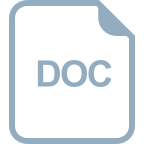
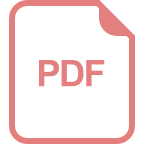

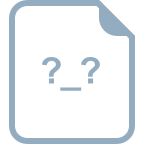
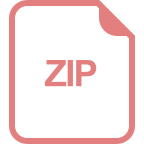
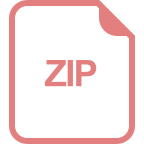
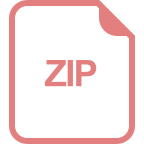
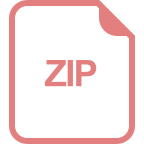
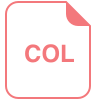
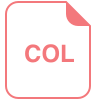
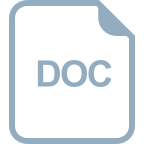
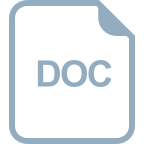
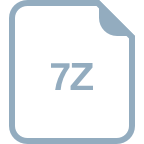
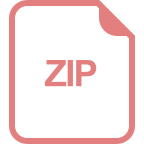
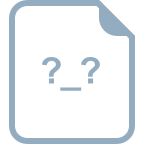
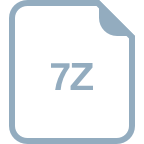