飞机大战游戏代码c++
时间: 2023-12-05 17:38:48 浏览: 67
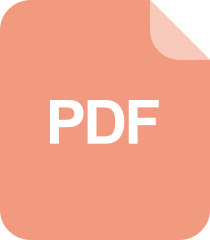
C++编写简易的飞机大战

以下是一个简单的C++飞机大战游戏代码,其中包括飞机的移动、子弹的发射、敌机的生成和碰撞检测等功能。代码中包含注释,方便理解。
```c++
#include <iostream>
#include <conio.h>
#include <windows.h>
#include <time.h>
using namespace std;
const int width = 40; // 游戏界面宽度
const int height = 20; // 游戏界面高度
int x, y; // 飞机的坐标
int score; // 得分
bool gameover; // 游戏是否结束
char map[height][width]; // 游戏界面
void init() { // 初始化游戏界面
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
if (i == 0 || i == height - 1 || j == 0 || j == width - 1) {
map[i][j] = '#'; // 边界用#表示
} else {
map[i][j] = ' '; // 其他地方为空格
}
}
}
x = width / 2; // 飞机初始位置在界面中央
y = height - 2;
map[y][x] = '^'; // 飞机用^表示
score = 0;
gameover = false;
}
void draw() { // 绘制游戏界面
system("cls"); // 清屏
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
cout << map[i][j];
}
cout << endl;
}
cout << "Score: " << score << endl;}
void input() { // 处理用户输入
if (_kbhit()) { // 如果有按键按下
switch (_getch()) {
case 'a': // 左移
if (x > 1) {
map[y][x] = ' ';
x--;
map[y][x] = '^';
}
break;
case 'd': // 右移
if (x < width - 2) {
map[y][x] = ' ';
x++;
map[y][x] = '^';
}
break;
case ' ': // 发射子弹
for (int i = y - 1; i >= 0; i--) {
if (map[i][x] == '*') { // 如果击中敌机
score++;
map[i][x] = ' ';
break;
} else if (map[i][x] == '#') { // 如果撞到边界
break;
} else {
map[i + 1][x] = ' ';
map[i][x] = '*';
}
}
break;
case 'q': // 退出游戏
gameover = true;
break;
}
}
}
void update() { // 更新游戏状态
// 移动敌机
for (int i = 1; i < height - 1; i++) {
for (int j = 1; j < width - 1; j++) {
if (map[i][j] == 'o') {
map[i][j] = ' ';
if (map[i + 1][j] == '^') { // 如果撞到飞机
gameover = true;
return;
} else if (map[i + 1][j] == '*') { // 如果被子弹击中
score++;
map[i + 1][j] = ' ';
} else if (map[i + 1][j] == ' ') {
map[i + 1][j] = 'o';
}
}
}
}
// 生成新的敌机
if (rand() % 10 == 0) {
int j = rand() % (width - 2) + 1;
if (map[1][j] == ' ') {
map[1][j] = 'o';
}
}
}
int main() {
srand(time(NULL)); // 初始化随机数种子
init(); // 初始化游戏界面
draw(); // 绘制游戏界面
while (!gameover) { // 游戏循环
input(); // 处理用户输入
update(); // 更新游戏状态
draw(); // 绘制游戏界面
Sleep(50); // 等待一段时间,降低游戏速度
}
cout << "Game Over!" << endl;
cout << "Your Score: " << score << endl;
return 0;
}
```
阅读全文
相关推荐
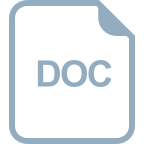
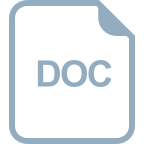

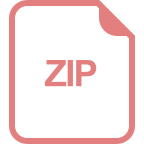
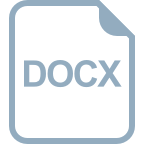
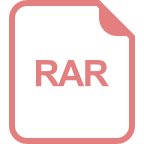
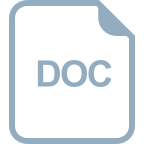
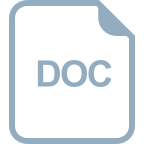





