mfc 动态改变已经生成的dialog对话框的大小
时间: 2023-07-16 11:15:50 浏览: 867
要动态改变已经生成的对话框的大小,可以使用以下步骤:
1. 打开对话框资源文件,双击对话框。在对话框设计器中,选择对话框的边框,然后将其属性中的“可调整大小”设置为“是”。
2. 在对话框类的头文件中添加以下成员变量:
```
int m_nWidth; // 对话框的宽度
int m_nHeight; // 对话框的高度
```
3. 在对话框类的 OnInitDialog 函数中,初始化成员变量 m_nWidth 和 m_nHeight 为对话框的初始宽度和高度。
4. 在对话框类中添加以下函数:
```
void SetDialogSize(int nWidth, int nHeight)
{
CRect rect;
GetWindowRect(&rect);
MoveWindow(rect.left, rect.top, nWidth, nHeight, TRUE);
}
```
此函数将对话框的宽度和高度设置为指定的值。
5. 当需要改变对话框的大小时,调用 SetDialogSize 函数,传入新的宽度和高度值即可。
```
SetDialogSize(500, 300); // 将对话框设置为宽度为 500,高度为 300
```
注意,如果对话框中包含子控件,改变对话框大小可能会导致控件位置和大小的变化。在更改对话框大小后,您可能需要手动调整子控件的位置和大小以适应新的对话框大小。
相关问题
mfc改变已经生成的dialog对话框的大小
要更改已经生成的 MFC 对话框的大小,可以执行以下步骤:
1. 打开您的 MFC 对话框项目并选择要更改大小的对话框。
2. 双击该对话框以打开其资源编辑器。
3. 在资源编辑器中,选择对话框并转到属性窗口。
4. 在属性窗口中,找到“Size”属性并更改它以反映所需的对话框大小。
5. 保存更改并重新生成您的项目。
6. 运行您的项目以查看对话框的新大小。
根据代码生成MFC对话框
将上述C++控制台应用程序转换为使用MFC(Microsoft Foundation Classes)的对话框应用程序涉及多个步骤。以下是一个简化的示例,展示如何将主要功能集成到一个MFC对话框应用程序中。
### 步骤概述
1. **创建MFC对话框项目**:使用Visual Studio创建一个新的MFC对话框应用程序。
2. **定义类**:将原有的`Course`和`Student`类定义移到MFC项目中。
3. **设计对话框界面**:在资源编辑器中设计对话框界面,包括按钮、文本框等控件。
4. **实现功能**:将原有逻辑迁移到相应的事件处理函数中。
### 示例代码
#### 1. 创建MFC对话框项目
打开Visual Studio,创建一个新的MFC应用程序项目,选择“基于对话框”模板。
#### 2. 定义类
在项目的头文件(例如`YourProjectNameDlg.h`)中定义`Course`和`Student`类:
```cpp
#pragma once
#include <unordered_map>
#include <vector>
#include <string>
class Course {
public:
std::string name;
int credits;
Course(const std::string& n, int c) : name(n), credits(c) {}
};
class Student {
public:
std::string id;
std::string className;
std::unordered_map<std::string, int> courseCredits;
Student(const std::string& nId = "", const std::string& nClassName = "",
const std::unordered_map<std::string, int>& nCourseCredits = {})
: id(nId), className(nClassName), courseCredits(nCourseCredits) {}
void addCourseCredit(const std::string& courseName, int credits) {
courseCredits[courseName] += credits;
}
int getTotalCredits() const {
int total = 0;
for (const auto& pair : courseCredits) {
total += pair.second;
}
return total;
}
void printCredits(CString& output) const {
output.Format(_T("学生ID: %s, 班级: %s\n学分信息:\n"), id.c_str(), className.c_str());
for (const auto& pair : courseCredits) {
output.AppendFormat(_T(" 课程: %s,学分: %d\n"), pair.first.c_str(), pair.second);
}
}
};
```
#### 3. 设计对话框界面
在资源编辑器中设计对话框界面,添加必要的控件,例如:
- 文本框用于输入学生ID、课程名称、学分等。
- 按钮用于触发各种操作(添加学分、查看学分、修改学分等)。
#### 4. 实现功能
在对话框类的实现文件(例如`YourProjectNameDlg.cpp`)中实现功能:
```cpp
#include "pch.h"
#include "framework.h"
#include "YourProjectName.h"
#include "YourProjectNameDlg.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#endif
// 全局变量存储学生信息
std::unordered_map<std::string, Student> students;
std::unordered_map<std::string, std::vector<std::string>> classStudents;
BEGIN_MESSAGE_MAP(CYourProjectNameDlg, CDialogEx)
ON_BN_CLICKED(IDC_ADD_CREDITS, &CYourProjectNameDlg::OnBnClickedAddCredits)
ON_BN_CLICKED(IDC_VIEW_STUDENT_CREDITS, &CYourProjectNameDlg::OnBnClickedViewStudentCredits)
ON_BN_CLICKED(IDC_VIEW_CLASS_CREDITS, &CYourProjectNameDlg::OnBnClickedViewClassCredits)
ON_BN_CLICKED(IDC_MODIFY_STUDENT_CREDITS, &CYourProjectNameDlg::OnBnClickedModifyStudentCredits)
ON_BN_CLICKED(IDC_SORT_BY_COURSE_CREDITS, &CYourProjectNameDlg::OnBnClickedSortByCourseCredits)
END_MESSAGE_MAP()
CYourProjectNameDlg::CYourProjectNameDlg(CWnd* pParent /*=nullptr*/)
: CDialogEx(IDD_YOURPROJECTNAME_DIALOG, pParent)
{
}
void CYourProjectNameDlg::DoDataExchange(CDataExchange* pDX)
{
CDialogEx::DoDataExchange(pDX);
}
BOOL CYourProjectNameDlg::OnInitDialog()
{
CDialogEx::OnInitDialog();
// 初始化数据
initializeData();
return TRUE; // return TRUE unless you set the focus to a control
// 异常: OCX 属性页应返回 FALSE
}
void CYourProjectNameDlg::OnBnClickedAddCredits()
{
CString studentID, courseName;
int credits;
GetDlgItemText(IDC_EDIT_STUDENT_ID, studentID);
GetDlgItemText(IDC_EDIT_COURSE_NAME, courseName);
GetDlgItemInt(IDC_EDIT_CREDITS, &credits);
auto it = students.find((LPCTSTR)studentID);
if (it != students.end()) {
it->second.addCourseCredit((LPCTSTR)courseName, credits);
MessageBox(_T("学分添加成功!"));
} else {
MessageBox(_T("未找到该学生,请先添加学生。"));
}
}
void CYourProjectNameDlg::OnBnClickedViewStudentCredits()
{
CString studentID, output;
GetDlgItemText(IDC_EDIT_STUDENT_ID, studentID);
auto it = students.find((LPCTSTR)studentID);
if (it != students.end()) {
it->second.printCredits(output);
SetDlgItemText(IDC_STATIC_OUTPUT, output);
} else {
MessageBox(_T("未找到该学生!"));
}
}
void CYourProjectNameDlg::OnBnClickedViewClassCredits()
{
CString className, output;
GetDlgItemText(IDC_EDIT_CLASS_NAME, className);
auto it = classStudents.find((LPCTSTR)className);
if (it != classStudents.end()) {
output.Format(_T("班级 %s 学生学分完成情况:\n"), (LPCTSTR)className);
for (const auto& studentID : it->second) {
auto studentIt = students.find((LPCTSTR)studentID);
if (studentIt != students.end()) {
studentIt->second.printCredits(output);
output.Append(_T("\n")); // 每个学生之间空一行
}
}
SetDlgItemText(IDC_STATIC_OUTPUT, output);
} else {
MessageBox(_T("未找到该班级!"));
}
}
void CYourProjectNameDlg::OnBnClickedModifyStudentCredits()
{
CString studentID, courseName;
int credits;
GetDlgItemText(IDC_EDIT_STUDENT_ID, studentID);
GetDlgItemText(IDC_EDIT_COURSE_NAME, courseName);
GetDlgItemInt(IDC_EDIT_CREDITS, &credits);
auto studentIt = students.find((LPCTSTR)studentID);
if (studentIt != students.end()) {
if (!courseName.IsEmpty()) {
// 查找当前课程的学分,如果不存在则默认为0
int currentCredits = 0;
auto courseIt = studentIt->second.courseCredits.find((LPCTSTR)courseName);
if (courseIt != studentIt->second.courseCredits.end()) {
currentCredits = courseIt->second;
}
// 更新学分(先减去旧的,再加上新的)
studentIt->second.courseCredits[(LPCTSTR)courseName] = credits - currentCredits + credits;
MessageBox(_T("学分修改成功!"));
} else {
studentIt->second.courseCredits.erase((LPCTSTR)courseName); // 删除该课程学分
MessageBox(_T("课程学分已删除!"));
}
} else {
MessageBox(_T("未找到该学生,请先添加学生。"));
}
}
void CYourProjectNameDlg::OnBnClickedSortByCourseCredits()
{
CString courseName, output;
GetDlgItemText(IDC_EDIT_COURSE_NAME, courseName);
std::vector<std::pair<std::string, int>> sortedCredits;
for (const auto& pair : students) {
auto it = pair.second.courseCredits.find((LPCTSTR)courseName);
if (it != pair.second.courseCredits.end()) {
sortedCredits.emplace_back(pair.first, it->second);
}
}
std::sort(sortedCredits.begin(), sortedCredits.end(),
[](const auto& a, const auto& b) { return a.second > b.second; });
output.Format(_T("按课程 %s 学分高低排序的学生列表:\n"), (LPCTSTR)courseName);
for (const auto& entry : sortedCredits) {
output.AppendFormat(_T("学生ID: %s, 学分: %d\n"), entry.first.c_str(), entry.second);
}
SetDlgItemText(IDC_STATIC_OUTPUT, output);
}
void initializeData() {
// 使用vector初始化一些学生数据
std::vector<std::pair<std::string, std::pair<std::string, std::unordered_map<std::string, int>>>> initialStudents = {
{"001", {"ClassA", {{"Basic", 20}, {"Specialized", 4}, {"Elective", 10}, {"Humanities", 8}, {"Practical", 20}}}},
{"002", {"ClassB", {{"Basic", 50}, {"Specialized", 3}, {"Elective", 24}, {"Humanities", 5}, {"Practical", 15}}}},
{"003", {"ClassA", {{"Basic", 40}, {"Specialized", 8}, {"Elective", 18}, {"Humanities", 4}, {"Practical", 18}}}},
{"004", {"ClassB", {{"Basic", 50}, {"Specialized", 6}, {"Elective", 20}, {"Humanities", 3}, {"Practical", 10}}}}
};
// 将初始数据添加到全局变量中
for (const auto& student : initialStudents) {
students[student.first] = Student{student.first, student.second.first, student.second.second};
classStudents[student.second.first].push_back(student.first);
}
}
```
### 总结
以上示例展示了如何将控制台应用程序的功能迁移到MFC对话框应用程序中。主要步骤包括定义类、设计对话框界面和实现功能。通过这种方式,你可以将现有的逻辑无缝集成到MFC框架中。
阅读全文
相关推荐





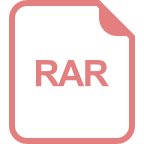










