题要求实现一个函数,实现三元组顺序表表示的稀疏矩阵转置。要c语言代码
时间: 2024-05-11 15:19:32 浏览: 149
以下是一个简单的实现:
```c
#include <stdio.h>
#define M 3 // 行数
#define N 4 // 列数
// 定义三元组结构体
typedef struct {
int row;
int col;
int val;
} Triple;
// 定义矩阵转置函数
void transpose(Triple a[], Triple b[]) {
int num[M] = {0}; // 存储每一行中非零元素个数
int cpot[M] = {0}; // 存储每一行中第一个非零元素的位置
for (int i = 0; i < M*N; i++) {
if (a[i].val != 0) {
int j = a[i].col;
num[j]++;
}
}
cpot[0] = 0;
for (int i = 1; i < M; i++) {
cpot[i] = cpot[i-1] + num[i-1];
}
for (int i = 0; i < M*N; i++) {
if (a[i].val != 0) {
int j = a[i].col;
int k = cpot[j];
b[k].row = a[i].col;
b[k].col = a[i].row;
b[k].val = a[i].val;
cpot[j]++;
}
}
}
// 测试函数
void printTriple(Triple a[], int len) {
for (int i = 0; i < len; i++) {
printf("(%d, %d, %d) ", a[i].row, a[i].col, a[i].val);
}
printf("\n");
}
int main() {
Triple a[M*N] = {
{0, 0, 1},
{0, 3, 3},
{1, 1, 2},
{2, 0, 4},
{2, 2, 5}
};
Triple b[M*N] = {0};
printf("原始矩阵:\n");
printTriple(a, M*N);
transpose(a, b);
printf("转置矩阵:\n");
printTriple(b, M*N);
return 0;
}
```
其中,`Triple` 结构体表示一个三元组,包含行号、列号和值。`transpose` 函数实现矩阵转置,输入为三元组顺序表 `a`,输出为三元组顺序表 `b`。`printTriple` 函数用于打印三元组。在 `main` 函数中,定义了一个大小为 3x4 的稀疏矩阵,调用 `transpose` 函数进行转置,并输出结果。
阅读全文
相关推荐
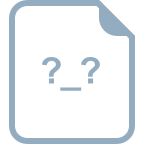
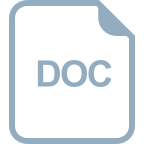
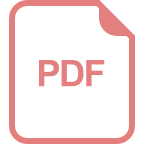















